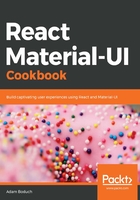
How it works...
This example defines three components, as follows:
- The MyToolbar component
- The MyDrawer component
- The main app component
Let's walk through each of these individually, starting with MyToolbar:
const MyToolbar = withStyles(styles)(
({ classes, title, onMenuClick }) => (
<Fragment>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
onClick={onMenuClick}
>
<MenuIcon />
</IconButton>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
{title}
</Typography>
</Toolbar>
</AppBar>
<div className={classes.toolbarMargin} />
</Fragment>
)
);
The MyToolbar component renders an AppBar component that accepts a title property and a onMenuClick() property. Both of these properties are used to interact with the MyDrawer component. The title property changes when a drawer item selection is made. The onMenuClick() function changes state in your main app component, causing the drawer to display. Next, let's take a look at MyDrawer:
const MyDrawer = withStyles(styles)(
({ classes, variant, open, onClose, setTitle }) => (
<Drawer variant={variant} open={open} onClose={onClose}>
<List>
<ListItem
button
onClick={() => {
setTitle('Home');
onClose();
}}
>
<ListItemText>Home</ListItemText>
</ListItem>
<ListItem
button
onClick={() => {
setTitle('Page 2');
onClose();
}}
>
<ListItemText>Page 2</ListItemText>
</ListItem>
<ListItem
button
onClick={() => {
setTitle('Page 3');
onClose();
}}
>
<ListItemText>Page 3</ListItemText>
</ListItem>
</List>
</Drawer>
)
);
The MyDrawer component is functional like MyToolbar. It accepts properties instead of maintaining its own state. For example, the open property is how the visibility of the drawer is controlled. The onClose() and setTitle() properties are functions that are called when drawer items are clicked on.
Finally, let's look at the app component where all of the state lives:
function AppBarInteraction({ classes }) {
const [drawer, setDrawer] = useState(false);
const [title, setTitle] = useState('Home');
const toggleDrawer = () => {
setDrawer(!drawer);
};
return (
<div className={classes.root}>
<MyToolbar title={title} onMenuClick={toggleDrawer} />
<MyDrawer
open={drawer}
onClose={toggleDrawer}
setTitle={setTitle}
/>
</div>
);
}
The title state is passed to the MyDrawer component, along with the toggleDrawer() function. The MyDrawer component is passed the drawer state to control visibility, the toggleDrawer() function to change visibility, and the setTitle() function to update the title in MyToolbar.