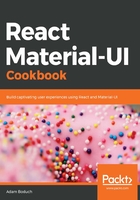
There's more...
What if you want the flexibility of having a persistent drawer that can be toggled using the same menu button in the App bar? Let's add a variant property to the AppBarInteraction component that is passed to MyDrawer. This can be changed from temporary to persistent and the menu button will still work as expected.
Here's what a persistent drawer looks like when you click on the menu button:

The drawer overlaps the App bar. Another problem is that if you click on any of the drawer items, the drawer is closed, which isn't ideal for a persistent drawer. Let's fix both of these issues.
First, let's address the z-index issue that's causing the drawer to appear on top of the App bar. You can create a CSS class that looks like this:
aboveDrawer: {
zIndex: theme.zIndex.drawer + 1
}
You can apply this class to the AppBar component in MyToolbar, as follows:
<AppBar className={classes.aboveDrawer}>
Now when you open the drawer, it appears underneath the AppBar, as expected:

Now you just have to fix the margin. When the drawer uses the persistent variant, you can add the toolbarMargin class to a <div> element as the first element in the Drawer component:
<div
className={clsx({
[classes.toolbarMargin]: variant === 'persistent'
})}
/>
With the help of the clsx() function, the toolbarMargin class is only added when needed – that is, when the drawer is persistent. Here's what it looks like now:

Lastly, let's fix the issue where the drawer closes when a drawer item is clicked on. In the main app component, you can add a new method that looks like the following code block:
const onItemClick = title => () => {
setTitle(title);
setDrawer(variant === 'temporary' ? false : drawer);
};
The onItemClick() function takes care of setting the text in the App bar, as well as closing the drawer if it's temporary. To use this new function, you can replace the setTitle property in MyDrawer with an onItemClick property. You can then use it in your list items, as follows:
<List>
<ListItem button onClick={onItemClick('Home')}>
<ListItemText>Home</ListItemText>
</ListItem>
<ListItem button onClick={onItemClick('Page 2')}>
<ListItemText>Page 2</ListItemText>
</ListItem>
<ListItem button onClick={onItemClick('Page 3')}>
<ListItemText>Page 3</ListItemText>
</ListItem>
</List>
Now when you click on items in the drawer when it's persistent, the drawer will stay open. The only way to close it is by clicking on the menu button beside the title in the App bar.