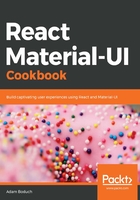
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have a Drawer component with a few items in it. You also have an AppBar component with a menu button and a title. The menu button should toggle the visibility of the drawer, and clicking on a drawer item should update the title in the AppBar. Here's the code to do it:
import React, { useState, Fragment } from 'react';
import { withStyles } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import Drawer from '@material-ui/core/Drawer';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemIcon from '@material-ui/core/ListItemIcon';
import ListItemText from '@material-ui/core/ListItemText';
import IconButton from '@material-ui/core/IconButton';
import MenuIcon from '@material-ui/icons/Menu';
const styles = theme => ({
root: {
flexGrow: 1
},
flex: {
flex: 1
},
menuButton: {
marginLeft: -12,
marginRight: 20
},
toolbarMargin: theme.mixins.toolbar
});
const MyToolbar = withStyles(styles)(
({ classes, title, onMenuClick }) => (
<Fragment>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
onClick={onMenuClick}
>
<MenuIcon />
</IconButton>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
{title}
</Typography>
</Toolbar>
</AppBar>
<div className={classes.toolbarMargin} />
</Fragment>
)
);
const MyDrawer = withStyles(styles)(
({ classes, variant, open, onClose, setTitle }) => (
<Drawer variant={variant} open={open} onClose={onClose}>
<List>
<ListItem
button
onClick={() => {
setTitle('Home');
onClose();
}}
>
<ListItemText>Home</ListItemText>
</ListItem>
<ListItem
button
onClick={() => {
setTitle('Page 2');
onClose();
}}
>
<ListItemText>Page 2</ListItemText>
</ListItem>
<ListItem
button
onClick={() => {
setTitle('Page 3');
onClose();
}}
>
<ListItemText>Page 3</ListItemText>
</ListItem>
</List>
</Drawer>
)
);
function AppBarInteraction({ classes }) {
const [drawer, setDrawer] = useState(false);
const [title, setTitle] = useState('Home');
const toggleDrawer = () => {
setDrawer(!drawer);
};
return (
<div className={classes.root}>
<MyToolbar title={title} onMenuClick={toggleDrawer} />
<MyDrawer
open={drawer}
onClose={toggleDrawer}
setTitle={setTitle}
/>
</div>
);
}
export default withStyles(styles)(AppBarInteraction);
Here's what the screen looks like when it first loads:

When you click on the menu icon button to the left of the title, you'll see the drawer:

If you click on the Page 2 item, the drawer will close and the title of the AppBar will change:
