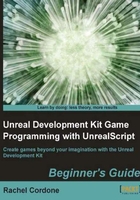
上QQ阅读APP看书,第一时间看更新
Time for action – Experiments with inheritance
Let's add a variable to our AwesomeGun
class and see how it works with another class we'll create.
- Add an int to our
AwesomeGun
class calledMyInt
. Our code should now look like this:class AwesomeGun extends UTWeap_RocketLauncher_Content; var int MyInt; defaultproperties { FireInterval(0)=0.1 ShotCost(0)=0 }
- Now create another class in our
AwesomeGame/Classes
folder calledAnotherGun.uc
. Type the following code into it: - Compile the code. We'll see that it compiles fine as our
AnotherGun
is inheritingMyInt
fromAwesomeGun
. - Now let's change the class we're extending from to be the same as AwesomeGun's parent class:
class AnotherGun extends UTWeap_RocketLauncher_Content; defaultproperties { MyInt=4 }
- Now when we compile, we'll get a warning:
Warning, Unknown property in defaults: MyInt=4
What just happened?
Even though the classes extend off of the same class, inheritance only happens when the class we want to use the variable in is inside the one that declares the variable in the class tree. We can change the default property of the variable for our class, and this is how we get different functionality out of them such as our example with the firing rate.