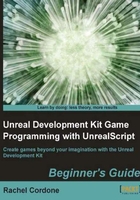
Time for action – Making a custom weapon
The best way to learn about inheritance is to see it in action, and the most basic way to see it is through a game's weapons. They're easy to modify and are a good starting point for learning about the UDK's classes.
- Create a new
.uc
file in ourAwesomeGame
/Classes
folder and call itAwesomeGun.uc
. Write the following code in it:class AwesomeGun extends UTWeap_RocketLauncher_Content; defaultproperties { FireInterval(0)=0.1 ShotCost(0)=0 }
- Compile our class. Now here's where we would ask, "How did it compile? I didn't declare any variables, but we're putting some in our default properties!" This is how inheritance works. We already saw the
ShotCost
variable inUTWeapon
on line 27:/** Holds the amount of ammo used for a given shot */ var array<int> ShotCost;
If we look higher up in the class tree at Weapon, we can see
FireInterval
on line 44 (as of the October 2011 build):/** Holds the amount of time a single shot takes */ var() Array<float> FireInterval;
When we create our class, any variables, properties, and functions of the classes higher in the tree are automatically created inside our class. This saves a lot of duplicated code, as anything that's going to be common to all of the subclasses only needs to be declared once. Remember when I said that a lot of programming is reading through the source code? This is why. To understand what functionality is already there and what variables we can already use, it's important to read through the classes higher up in the tree to see what they can do. This also prevents us from reinventing the wheel as it were, writing code to do something that already exists.
In our case, using the already existing
FireInterval
andShotCost
keeps us from having to write any code at all to change the way our gun works. We can just change the default properties in our class. - Open up the editor. To use our new weapon, we're going to need to place a weapon factory. In the Actor Classes browser, make sure Categories is unchecked, then browse down to NavigationPoint | PickupFactory | UDKPickupFactory | UTPickupFactory | UTWeaponPickupFactory. Place a UTWeaponPickupFactory on the floor where our AwesomeActors are, and delete our AwesomeActors.
- Double-click on the factory to open its properties, and change its Weapon Pickup Class to our AwesomeGun.
- One minor thing to do, unrelated to our programming. Since the weapon factory we placed is a navigation point, we need to rebuild paths in the editor to prevent us from getting warnings about it when we open up the map again later. Click on the build paths icon in the top toolbar, and then close the window that comes up afterwards.
- Save the map and test it out. Run over to the weapon factory to pick up our custom gun, and then spray the level down with rockets.
What just happened?
Boosh! And/or kakow! The changes we made were simple, but we can easily see how they affected the game. Changing the ShotCost
to 0
effectively gave us infinite ammo, since firing a rocket consumes 0 ammo. Changing the FireInterval
to 0.1
made it so that we fire ten rockets per second.
It's important to remember that variables and functions that are inherited only come from classes directly above ours in the class tree. As an experiment, let's create a subclass of our AwesomeGun
.