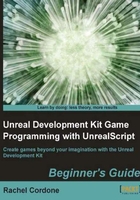
Time for action – Using integers
Let's make an Int.
- Declaring an integer is similar to what we did with bools, so let's replace our bool declaration line with this:
var int NumberOfKittens;
We can see that we have the same
var
text that declares our variable, and then we tell the game that our variable is anint
. Finally, we set the name of ourint
toNumberOfKittens
.The name of the variable should give a hint as to the difference between ints and floats, and why we need ints to begin with instead of using floats for everything. Since we don't want to hurt any defenseless kittens we should only be using whole numbers to represent the number of them. We don't want to have half of a kitten.
- As with our bool variable ints have a default value, in this case zero. We can check this by changing our
PostBeginPlay
function:function PostBeginPlay() { 'log("Number of kittens:" @ NumberOfKittens); }
Now our
AwesomeActor.uc
class should look like this:class AwesomeActor extends Actor placeable; var int NumberOfKittens; function PostBeginPlay() { 'log("Number of kittens:" @ NumberOfKittens); } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' HiddenGame=True End Object Components.Add(Sprite) }
- Let's compile and run the game to test it out!
[0007.63] ScriptLog: Number of kittens: 0
Notice there is no decimal place after the
0
; this is what makes an int an int. We use this type of variable for things that wouldn't have fractions, like the number of kills a player has made in deathmatch or the number of people in a vehicle. - Now let's see what happens when we change the variable. At the beginning of our
PostBeginPlay
function add this:NumberOfKittens = 5;
Now the function should look like this:
function PostBeginPlay() { NumberOfKittens = 5; 'log("Number of kittens:" @ NumberOfKittens); }
- Let's compile and test!
[0008.07] ScriptLog: Number of kittens: 5
- What would happen if we tried to add a fraction to our int? Only one way to find out! Change the line to this and compile:
NumberOfKittens = 5.7;
- Well, it compiles, so the engine obviously doesn't care about that
.7
of a kitten, but what actually happens? Run the game and then check the log to find out.[0007.99] ScriptLog: Number of kittens: 5
Interesting! We can see that not only did it ignore the fraction, but also truncated it instead of trying to round it up to 6. This is important behavior to remember about ints. Ints will also act this way when we use math on them.
- Change the line to this:
NumberOfKittens = 10 / 3;
This should end up as 3.333333, but with the truncation, we can see that it ignores the fraction.
[0007.72] ScriptLog: Number of kittens: 3
What just happened?
Ints are one of the ways the game stores numbers, and we use it when we don't need to worry about fractions. Usually we use them when we're just trying to count something and not trying to perform complex math with them. For that, we would use floats. Let's take a look at those now.