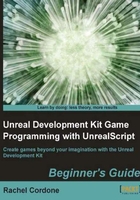
Time for action – Using booleans
The first thing we need to do is tell the game about our variable. This is called declaration. Variables need to be declared before they can be used. Our declaration tells the game what type of variable it is as well as its name.
We'll continue with our "is it raining?" scenario for this experiment. In a game we might want to use this variable to check whether we should spawn rain effects or make changes to the lights, and so on.
Variable declaration in UnrealScript happens after the class declaration line, and before any functions. Let's add a variable to AwesomeActor.uc
to see if it's raining or not.
- Open up our
AwesomeActor.uc
class in ConTEXT and add this line after our class declaration:var bool bIsItRaining;
The
var
tells the game that we're declaring a variable. After that, is the variable type, in this casebool
for boolean. After that, we tell the game our variable's name,bIsItRaining
. Spaces can't be used in variable names, but underscore characters (_
) are allowed. The semicolon finishes the line. It's important never to forget the semicolon at the end of the line. Without it the compiler will think any lines after it are part of this line and will look at us confused, as well as give us an error. - Now let's add a log to our
PostBeginPlay
function to see our variable in action. Change thePostBeginPlay
function to this (don't forget the tilde):function PostBeginPlay() { 'log("Is it raining?" @ bIsItRaining); }
This will output our text as well as tell us the value of
bIsItRaining
. The@
operator combines the text with the value of the variable into one sentence which the log uses. This is known as concatenation and will be discussed later in the chapter.Our
AwesomeActor
class should look like this now:class AwesomeActor extends Actor placeable; var bool bIsItRaining; function PostBeginPlay() { 'log("Is it raining?" @ bIsItRaining); } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' HiddenGame=True End Object Components.Add(Sprite) }
- Now let's compile it. If we get any errors go back and make sure everything is spelled correctly and that we haven't missed any semicolons at the end of lines.
Open the editor and open our test map with the AwesomeActor placed, and run the game. Nothing obvious will happen, but let's close the game and see what our
Launch2.log
file looks like:[0008.59] ScriptLog: Is it raining? False
Our variable is working! As we can see, even without doing anything to it our variable has a default value. When created, booleans automatically start out false. This is a good thing to know when creating variables, especially booleans. It's best to avoid words like Not or No in boolean names to avoid having double negatives. For example, if we had a
bool
namedbIsNotActive
, and it was False, would the object be active or not? In this case it would be active, but to avoid confusion it would be better to have a variable namedbIsActive
so it would be easier to tell what it means when it's true or false. - Now that we have our bool, how do we change it? Let's add a line to our
PostBeginPlay
function.bIsItRaining = true;
Now our function should look like this:
function PostBeginPlay() { bIsItRaining = true; 'log("Is it raining?" @ bIsItRaining); }
- Compile and run the game again, and we should see the change in the log:
[0007.68] ScriptLog: Is it raining? True
There we go!
- We can also change it back just as easily. Let's add a line after our log to change it to false, and then add another log to see the change.
bIsItRaining = false; 'log("Is it raining?" @ bIsItRaining);
Now our PostBeginPlay function should look like this:
function PostBeginPlay() { bIsItRaining = true; 'log("Is it raining?" @ bIsItRaining); bIsItRaining = false; 'log("Is it raining?" @ bIsItRaining); }
- Let's compile and test out the changes!
[0007.65] ScriptLog: Is it raining? True [0007.65] ScriptLog: Is it raining? False
What just happened?
There isn't much to use in booleans; they're the simplest type of variable in UnrealScript. Don't underestimate them though, they may be simple, but a lot of the variables we'll be working with will be bools. Anything where we only need a simple true/false answer will fall under this category.
Integers and floats
Next in our tour of UnrealScript variables are integers and floats. Both store numbers, but the difference is that integers (int for short) store whole numbers without a decimal point like 12, while floats can store fractions of numbers, like 12.3. Let's take a look at how to use them.