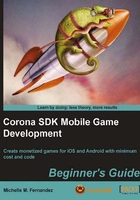
Optimizing your workflow
So far, we have touched on the vital basics of programming in Lua and terminology used in Corona SDK. Once you start developing interactive applications to sell in the App Store or Google Play Store, you need to be aware of your design choices and how they affect the performance of your application. This means taking into consideration how much memory your mobile device is using to process the application. Here are some things to look for if you're just starting out on Corona SDK.
Using memory efficiently
In some of our earlier examples, we used global variables in our code. Cases like those are an exception since the examples did not contain a high volume of functions, loops to call out to or display objects. Once you start building a game that is heavily involved with function calls and numerous display objects, local variables will increase performance within your application and will be placed on the stack so Lua can interface with them faster.
The following code will cause memory leaks:
-- myImage is a global variable
myImage = display.newImage( "image.png" )
myImage.x = 160; myImage.y = 240
-- A touch listener to remove object
local removeBody = function( event )
local t = event.target
local phase = event.phase
if "began" == phase then
-- variable "myImage" still exists even if it's not displayed
t:removeSelf() -- Destroy object
end
-- Stop further propagation of touch event
return true
end
myImage:addEventListener( "touch", removeBody )
The preceding code removes myImage
from the display hierarchy once it is touched. The only problem is that the memory used by myImage
leaks because the variable myImage
still refers to it. Since myImage
is a global variable, the display object it references will not be freed even though myImage
does not display on the screen.
Unlike global variables, localizing variables help speed up the look-up process for your display object. Also, it only exists within the block or chunk of code that it's defined in. Using a local variable in the following code will remove the object completely and free up memory:
-- myImage is a local variable
local myImage = display.newImage( "image.png" )
myImage.x = 160; myImage.y = 240
-- A touch listener to remove object
local removeBody = function( event )
local t = event.target
local phase = event.phase
if "began" == phase then
t:removeSelf() -- Destroy object
end
-- Stop further propagation of touch event
return true
end
myImage:addEventListener( "touch", removeBody )
Optimizing your display images
It's important to optimize your image file size as much as you can. Using full-screen images can impact the performance of your application. They require more time to load on a device and consume a lot of texture memory. When a lot of memory is consumed in an application, in most cases it'll be forced to quit.
iOS devices vary in available memory, depending which one you have:
- iPhone 3G, iTouch 2G—128 MB RAM
- iPhone 3GS, iPad, iTouch 3G/4G—256 MB RAM
- iPhone 4/4S, iPad 2—512 MB RAM
For example, texture memory on the iPhone 3GS should be kept under 25 MB before performance issues start occurring by slowing down your app or even forcing it to quit. An iPad 2 would have no problem going further downthat boundary since it has more memory available.
Refer to the following link to apply memory warnings for iOS devices: http://developer.anscamobile.com/reference/index/memorywarning-ios.
For Android devices, there is around a 24 MB memory limit. So it's important to be aware of how many display objects you have in your scene and how to manage them when they are not needed anymore in your app.
In cases where you no longer need an image to be displayed on screen use the following code:
image.parent:remove( image ) -- remove image from hierarchy -- or -- image:removeSelf( ) -- same as above
If you want to remove an image from the scene completely throughout the lifetime of your app, include the following line after your image.parent:remove( image )
or image:removeSelf()
code:
image = nil
Keeping memory usage low within your application will prevent crashes and improve performance. For more information on optimization, go to the following URL: http://developer.anscamobile.com/content/performance-and-optimization.
Pop quiz – basics of Lua
- Which of the following are values?
a. Numbers
b. nil
c. Strings
d. All of the above
- Which relational operator is false?
a.
print(0 == 0)
b.
print(3 >= 2)
c.
print(2 ~= 2)
d.
print(0 ~= 2)
- What is the correct way to scale an object in the x direction?
a.
object.scaleX
b.
object.xscale
c.
object.Xscale
d.
object.xScale