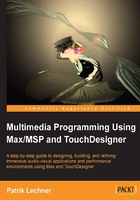
Creating our Hello World program
So let's start up Max, close the splash screen if you haven't got rid of it already, and make a new patch (command/Ctrl + N). You will see something like what's shown in the following screenshot; without the objects, the cords, the message box, and the sidebar might not be open or it might be in another mode. You don't need to open the sidebar for now, but if you like it, press the button with the number 11 in the following screenshot. We can have the Max window in a separate window. Either way, free floating or in the side bar, open it and I highly recommend that you remember the shortcut for it, which is command/Ctrl + M. Now, we have everything set up to write the obligatory Hello World program:

So this is our Hello World program. How was this created? What do we see here?
Dissection and construction
First of all, we see three objects here. A [button]
, a [message]
, and a [print]
object (and of course, a comment
object that reads click here!). So we can create them just like that; we create a new object in the patch by just hitting n and start writing. In one object, we write button
; in the second one, we write message
; and in the third one, we write print
. The purpose of this little patch is obviously to print the Hello World message to the Max window, which in this case is attached and not a separate window. Then, we interconnect these objects.
Now, here is the ninja way to do it:
- Make a new patcher: (command/Ctrl + N).
- Hit B to get the button right away.
- Hit M to get a message box and position the message box below the button object using arrow keys on the keyboard. Use Shift + arrow keys for momentary toggling snap to grid on and off. Hit Enter again to edit the contents of the message box and hit Shift + Enter to finish editing it.
- Hit N for a new object, type
print
, hit Enter, and position it below. - If you want to get the selection back on another object to edit or reposition it again, you can use command/Ctrl + the arrow keys.
- Now, if you have the max toolbox (mentioned in the externals section) installed, select them all and hit Shift + C. Otherwise, just connect them manually, and we are done! Now, this sounds pretty complicated, and you don't have to remember all these shortcuts, but if you know some of them, you'll have a better time coding with Max, I promise. Just try it out, it's not so hard!
Tip
There are two files that mainly define our keyboard shortcuts: Max 6.1/Cycling '74/interfaces/maxinterface.json
and Max 6.1/Cycling '74/init/max-keycommands.txt
.
Be sure to take a backup when redefining things there.
If you try out your patcher, you might need to Lock the patcher before the button reacts to your clicks in the expected way. In Max, there are two modes of interaction with your patcher: the edit mode and the locked mode. Naturally, one is for editing your program and one is for actual interaction. So in order to be able to click on the button, we have four ways to enter the locked mode:
- Clicking on the little lock icon on the bottom left of the patcher window
- Using the shortcut command/Ctrl + E to toggle between the modes
- Using command/Ctrl + left mouse button to click into an empty area of the patcher to toggle the modes
- Pressing and holding command/Ctrl during the interaction with the GUI
The last option is the preferred one during development since one often needs to only adjust one value or quickly click a button, but actually, one is still patching and not performing.
Contents
Let's think about the actual contents of this patcher. We have a user interface object, namely a button. We have a message box and the print
object. If we click on the button, it actually sends a message out of its outlet, called a bang message. We discussed this in Chapter 1, Getting Started with Max. It tells the next object to act now and to do whatever it is supposed to do, nearly always to output data.
Note
Exercise
Prove that the message that goes from the button to the message box actually is a bang message.
The [print] object
Before we go over the message box, there are a couple of things we need to go over in relation to the print
object. When programming with Max, we must always remember that print
is one of our best friends. As in many other programming languages, there are various ways to output data or debug processes, but the print
statement/object is at the heart of debugging. Whenever we aren't sure what's happening, we put print
objects everywhere.
Note
Caution
Printing a lot to the max window drains more performance than you might think! Keep track of your print
objects that lie around and consider using mechanisms such as [gate]
and the [send]
/[receive]
system to deactivate printing globally.
The print
object takes an argument that will show up in the Max window's object column instead of print, so we can design our printouts to be a bit more informative, as in the following screenshot. Also, if we double-click on a line in the Max window, Max highlights the corresponding print object.
Note
Many beginners struggle with errors in their patchers although Max is actually telling them what's wrong or at least gives a hint. Max also communicates with us through the Max window (not only print
objects), so keep an eye on it. A typical window layout to do standard work in Max has a patcher window, an Inspector window, and the Max window open and visible.
Look at the following screenshot to see an example of printing:

The debugging facilities of printing might also be the reason why the Hello World program is so popular for starting to program in a specific language. We will need a simple debugging tool right at the beginning, so we can always see what we are doing. Debugging is not the most exciting topic in the world and nobody wants to learn a lot about how to treat the mistakes before starting to do anything. This book contains a section on debugging, and I invite you to refer to it whenever you feel the need for it. We'll cover this rather late and you might want to avoid the situation in which you say "Well, great, why didn't you tell me this earlier?"
The message box
The message box
object is a very versatile special object. You will find it everywhere, and while we will use it in various ways in this book, not all of its functionality is covered here. As you know, you can always get help if you press Alt + click on an object. Here are some of its main features:
- We can edit the content and write any message in there that we would want to send anywhere.
- We can set the contents of it dynamically by sending anything into the right inlet.
- We can, as you have seen, send out the contents by sending a bang into its left inlet.
- Moreover, if we click on it (when in locked mode), it also sends out its contents, so it's also a basic text button.
You see it's an object that helps us in many situations and the following screenshot shows of some more advanced features of it. We can use it for the basic formatting of messages and lists and even ordering things in time.

Using the $n
object (where n is a number between 1 and 9) notation enables us to generate compound messages on the fly as you can see. Also, the use of commas is important to notice here. Commas divide the contents up into several messages in sequence. These messages are sent in sequence, as fast as Max can.
Since we saw that we can display messages using the message box right inlet, it's also a good debugging tool. However, the patcher shown in the previous screenshot shows a subtle difference between print
and the message box
object. Look at the right-hand section where we used the commas. The message box
object used for display only shows the sequence. That's not because this is a screenshot; try it out, you won't see the other messages (Max is too fast!). In contrast to that, [print]
can be used very well for this task; all messages appear in chronological order (by default at least).
The MSP-Hello World
By now, you should be able read data from any point in Max using either the message box
or the print
statement. But what about streams of numbers and signals? What about MSP? Let's have a look at how to get information about an unknown signal. Depending on if it's a stream of Max messages or MSP signals, we are going to need different tools, as shown in the following screenshot:

In this screenshot, you can see patcher wysiwyg.maxpat
. Open it and play around with it. Don't look into the blackbox too soon (by double clicking it)! It generates two signals: one in the max domain, a stream of numbers of course, and the other one in the MSP domain. This patch may contain a lot of objects that are new to you, but you can just Alt + click on each of them to get to know them. The main point here is again to understand what we are doing. Although a signal has a lot of properties, often, the most important thing about an audio signal is how it sounds; as obvious as this sounds, don't forget this.
However, there are many other features a signal may have and many ways of looking at those. Take amplitude, for example; you can look at the numerical value, you can measure in dB or linear, or you can look at the peak value if you want to check whether your signal is clipping or use RMS, if you rather care for a rough guide of loudness. There are a number of new standards since the AES/EBU recommendation R128 that also describes properties of an audio signal that might be even more important than peak or RMS (namely LU, true peak, and dynamic range measurements).
All these more or less describe the amplitude of a signal and there are many more (for example, different frequency weighted measurements). Often, we care a lot more about the spectrum of a signal, hence the amplitudes of a signal's components, or even more sophisticated features of a signal.
The most important thing is to discover what we really want to know about a signal and how to get this information.
Note
Exercise
With the given wysiwyg.maxpat
patch, look at all the tools, get to know them, and do some research if you feel you don't understand a concept behind a measurement. Before looking into the blackbox, try to guess what's in there and build what you think might be in there, or draw a block diagram!