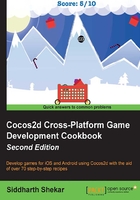
Adding a Gameplay Scene
After the play button is pressed, to actually transition to another scene, we need a new scene to create a transition into. So, let's take a look at how to create one.
Getting ready
Let's us now add a gameplay scene. Creating the file is similar to how we created it in the previous chapter.
How to do it…
Here, I created a class called GameplayScene
. The Gameplay.h
file should be as follows:
#import "CCScene.h" @interface GameplayScene : CCNode +(CCScene*)scene; -(id)initWithLevel:(NSString*)lvlNum @end
The GameplayScene.m
file should be as follows:
#import "GameplayScene.h" #import "cocos2d-ui.h" @implementation GameplayScene +(CCScene*)scene{ return[[self alloc]initWithLevel:lvlNum]; } -(id)initWithLevel:(NSString*)lvlNum{ if(self = [super init]){ CGSize winSize = [[CCDirector sharedDirector]viewSize]; //Basic CCSprite - Background Image CCSprite* backgroundImage = [CCSprite spriteWithImageNamed:@"Bg.png"]; backgroundImage.position = CGPointMake(winSize.width/2, winSize.height/2); [self addChild:backgroundImage]; CCLabelTTF *mainmenuLabel = [CCLabelTTF labelWithString:@"GameplayScene" fontName:@"AmericanTypewriter-Bold" fontSize: 36.0f]; mainmenuLabel.position = CGPointMake(winSize.width/2, winSize.height * 0.8); self addChild:mainmenuLabel]; CCLabelTTF *levelNumLabel = [CCLabelTTF labelWithString:lvlNum fontName:@"AmericanTypewriter-Bold" fontSize: 24.0f]; levelNumLabel.position = CGPointMake(winSize.width/2, winSize.height * 0.7); [self addChild:levelNumLabel]; CCButton *resetBtn = [CCButton buttonWithTitle:nil spriteFrame:[CCSpriteFrame frameWithImageNamed:@"resetBtn_normal.png"] highlightedSpriteFrame:[CCSpriteFrame frameWithImageNamed:@"resetBtn_pressed.png"] disabledSpriteFrame:nil]; [resetBtn setTarget:self selector:@selector(resetBtnPressed:)]; CCLayoutBox * btnMenu; btnMenu = [[CCLayoutBox alloc] init]; btnMenu.anchorPoint = ccp(0.5f, 0.5f); btnMenu.position = CGPointMake(winSize.width/2, winSize.height * 0.5); [btnMenu addChild:resetBtn]; [self addChild:btnMenu]; } return self; } -(void)resetBtnPressed:(id)sender{ CCLOG(@"reset button pressed"); } @end
How it works…
The GameplayScene
class is similar to MainScene
, except that we added a custom scene and init
function so that we can pass in a level number as a string to the class.
We also added a new label that will show the current level loaded.
The class, as such, doesn't do anything, but in the next section, we will take a look at how we can transition to the gameplay scene, where it will show which level we have currently loaded.