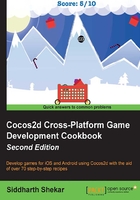
Adding buttons with CCMenu
Buttons are the primary way to navigate between different scenes. In this section, we will take a look at how to add buttons to the scene.
Getting ready
Buttons are also similar to regular sprites. In the case of buttons, we need two images. One image is shown when the button is normal, which means that it is not pressed, and the other image is shown when the button is pressed.
For this section, the resources are provided in the resources folder of this chapter. There are four images in total for the iPad and iPad HD—two for normal mode and two for button pressed. Copy these four files in the Resource/Publishes-iOS
folder on your system.
Also, add the following code at the top of the MenuScene.m
file to import the Cocos2d-ui.h
file. This is required to add the buttons and layout classes:
#import "cocos2d-ui.h"
How to do it…
Right after where we added the outline in the previous section, we will add the following code:
CCButton *playBtn = [CCButton buttonWithTitle:nil spriteFrame:[CCSpriteFrame frameWithImageNamed:@"playBtn_normal.png"] highlightedSpriteFrame:[CCSpriteFrame frameWithImageNamed:@"playBtn_pressed.png"] disabledSpriteFrame:nil];
To create buttons, we will have to use the CCButton
class. This will take four parameters: the title of the button, a spriteFrame
parameter for the normal mode, a second sprite frame for the highlighted or pressed mode, and a third sprite frame for the disabled mode.
For this example, we will just pass in the image for the normal and pressed modes.
If we run the project now, we will not see anything on the screen; this is because we have not yet added the buttons to the scene. To add buttons to a scene, we need to first add them to a button menu of the CCLayout
type and then to the scene.
So, let's create a new variable of the CCLayout
type next, as follows:
CCLayoutBox * btnMenu; btnMenu = [[CCLayoutBox alloc] init]; btnMenu.anchorPoint = ccp(0.5f, 0.5f); btnMenu.position = CGPointMake(winSize.width/2, winSize.height * 0.5); [btnMenu addChild:playBtn]; [self addChild:btnMenu];
We will create a new variable called btnMenu
of the CCLayout
type and then allocate and initiate the variable. Next, we will set the anchor point to center
because all the buttons will be anchored to the lower-left of the layout otherwise. The layout is then placed at the center of the screen.
Finally, we will add playBtn
as a child of btnMenu
and then add btnMenu
itself to the scene.

Now, we can see the button displayed on the scene. Also, if we press the button, it will change to the pressed image.
For the button to do something, we need to call a function once the button is pressed and released.
We will add the following highlighted code right after the playBtn
variable:
CCButton *playBtn = [CCButton buttonWithTitle:nil
spriteFrame:[CCSpriteFrame frameWithImageNamed:@"playBtn_normal.png"]
highlightedSpriteFrame:[CCSpriteFrame frameWithImageNamed:@"playBtn_pressed.png"]
disabledSpriteFrame:nil];
[playBtn setTarget:self selector:@selector(playBtnPressed:)];
Next, we will add the following code, which will get called when the button is pressed and released:
-(void)playBtnPressed:(id)sender{ CCLOG(@"play button pressed"); }
How it works…
We will assign the playBtnPressed
function to playBtn
. When the button is pressed and released, the function will get called. As of now, we will only log out a text that will tell us that the button is pressed in the console.
Press and release the play button to the see the console light up with the following text:
