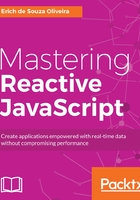
Subscribing using the log method
So far, every time we created an observable, we used the onValue() method to listen to events in the observable. In our examples, we usually just printed the value to the console,as this is common usage when testing observables and operators bacon.js has a special method through which you can print all the events to the console. All observables have the log() method. This method prints every event to the console and prints the <end> string when the event stream finishes. We can use it with EventStreams:
Bacon
.fromArray([1,2,3,4,5])
.log();
This code gives you the following output to the console:
1
2
3
4
5
<end>
As you can see, after all the events, it will print the <end> string to indicate the end of the EventStream. If we decide to use it with an infinite stream (a stream created with the interval() method), it will never print the <end> string (as you should expect). Refer to the following code:
Bacon
.interval(100)
.log()
This will print the following output:
{}
{}
{}
{}
It will keep on printing until you close the program.
We can also use the log() method with a Property, as you can see in the following example:
var stringProperty = Bacon
.fromArray(['a','b','c','d'])
.scan('=> ',(acc,b)=> acc+b);
stringProperty.log();
This will print the following output:
=>
=> a
=> ab
=> abc
=> abcd
<end>
As you can see, this prints the <end> string for a Property as well.