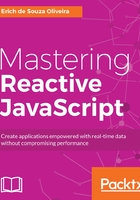
Subscribing using the onValue() method
The most common way of subscribing to an observable is using the onValue() method. This method has the following signature:
observable.onValue(functionToBeCalledWhenAnEventOccurs);
So let's subscribe to eventStream to log every event on this stream, as follows:
Bacon
.fromArray([1,2,3,4,5])
.onValue((number)=>console.log(number));
This code gives you the following output to the console:
1
2
3
4
5
This function can be used for any type of observable (EventStream and Property). The only difference is that in Properties, if the initial value of the Property exists, then it triggers the onValue() function. Check out the following code:
var initialValue =0;
Bacon
.fromArray([1,2,3,4,5])
.toProperty(initialValue)
.onValue((number)=>console.log(number));
This will give you the following output:
0
1
2
3
4
5
When subscribing to an observable, it's important you know a way to unsubscribe it as well. The onValue() method returns a function; this function when called will unsubscribe your function from this observable. So we can create an observable from an interval and print a message every time an event is propagated, as follows:
Bacon
.interval(1000)
.onValue(()=>(console.log("event happened")));
It will print the message event happened every second until we kill the process. But if we want, we can use the return of onValue() to unsubscribe from our observable after a certain amount of time, as follows:
var unsubscribe = Bacon
.interval(1000)
.onValue(()=>(console.log("event happened")));
setTimeout(function(){
console.log("unsubscribing")
unsubscribe();
},4000);
With this code, the program will unsubscribe from your observable. This way, it exits normally and prints the following output:
event happened
event happened
event happened
unsubscribing