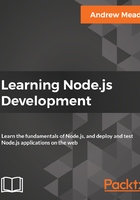
Dealing with the errors in parsing commands
Getting an error is not the end of the world. Getting an error usually means that you have a small typo or you forgot one step in the process. So, we'll first figure out how to parse through these error messages, because the error messages you get in the code output can be pretty daunting. Let's refer to the code output error here:

As you can see, the first line shows you where the error occurred. It's inside of our app.js file, and the number 19 after the colon is the line number. It shows you exactly where things went bad. The TypeError: notes.getNote is not a function line is telling you pretty clearly that the getNote function you tried to run doesn't exist. Now we can take this information and debug our app.
In app.js, we see that we call notes.getNote. Everything looks great, but when we move into notes.js, we realize that we never actually exported getNote. This is why when we try to call the function, we get getNote is not a function. All we have to do to fix that error message is export getNote, as shown here:
module.exports = {
addNote,
getAll,
getNote
};
Now when we save the file and rerun the app from Terminal, we'll get what we expect—Getting note followed by the title, which is accounts, as shown here:

This is how we can debug our error messages. Error messages contain really useful information. For the most part, the first couple of lines are code that you've written, and the other ones are internal Node code or third-party modules. In our case, the first line of the stack trace is important, as it shows exactly where the error occurred.