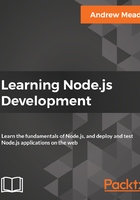
The read command
When the read command is used, we want to call notes.getNote, passing in title. Now, title will get passed in and parsed using yargs, which means that we can use argv.title to fetch it. And that's all we have to do when it comes to calling the function:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
const argv = yargs.argv;
var command = process.argv[2];
console.log('Command:', command);
console.log('Yargs', argv);
if (command === 'add') {
notes.addNote(argv.title, argv.body);
} else if (command === 'list') {
notes.getAll();
} else if (command === 'read') {
notes.getNote(argv.title);
} else if (command === 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
The next step is to define getNote, because currently it doesn't exist. Over in notes.js, right below the getAll variable, we can make a variable called getNote, which will be a function. We'll use the arrow function, and it will take an argument; it will take the note title. The getNote function takes the title, then it returns the body for that note:
var getNote = (title) => {
};
Inside getNote, we can use console.log to print something like Getting note, followed by the title of the note you will fetch, which will be the second argument to console.log:
var getNote = (title) => {
console.log('Getting note', title);
};
This is the first command, and we can now test it before we go on to the second one, which is remove.
Over in Terminal, we can use node app.js to run the file. We'll be using the new read command, passing in a title flag. I'll use a different syntax, where title gets set equal to the value outside of quotes. I'll use something like accounts:
node app.js read --title accounts
This accounts value will read the accounts note in the future, and it will print it to the screen, as shown here:

As you can see in the preceding code output, we get an error, which we'll debug now.