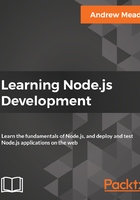
Working with the add command
Let's work with the add command, for example, for parsing your arguments and calling the functions. Once the add command gets called, we want to call a function defined in notes, which will be responsible for actually adding the note. The notes.addNote function will get the job done. Now, what do we want to pass to the addNote function? We want to pass in two things: the title, which is accessible on argv.title, as we saw in the preceding example; and the body, argv.body:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
const argv = yargs.argv;
var command = process.argv[2];
console.log('Command:', command);
console.log('Process', process.argv);
console.log('Yargs', argv);
if (command === 'add') {
console.log('Adding new note');
notes.addNote(argv.title, argv.body);
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else if (command === 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
Now that we have notes.addNote in place, we can remove our console.log statement, which was just a placeholder, and we can move into the notes application notes.js.
Inside notes.js, we'll get started by making a variable with the same name as the method we used over app.js and addNote, and we will set it equal to an anonymous arrow function, as shown here:
var addNote = () => {
};
Now, this alone isn't too useful, because we're not exporting the addNote function. Below the variable, we can define module.exports in a slightly different way. In previous sections, we added properties onto exports to export them. We can actually define an entire object that gets set to exports, and in this case, we can set addNote equal to the addNote function defined in preceding code block:
module.exports = {
addNote: addNote
};
In the preceding code, we're setting an object equal to module.exports, and that object has a property, addNote, which points to the addNote function we defined as a variable in the preceding code block.
Once again, addNote: and addNote are identical inside of ES6. We will be using the ES6 syntax for everything throughout this book.
Now I can take my two arguments, title and body, and actually do something with them. In this case, we'll call console.log and Adding note, passing in the two arguments as the second and third argument to console.log, title and body, as shown here:
var addNote = (title, body) => {
console.log('Adding note', title, body);
};
Now we're in a pretty good position to run the add command with title and body and see if we get exactly what we'd expect, which is the console.log statement shown in the preceding code to print.
Over in Terminal, we can start by running the app with node app.js, and then specify the filename. We'll use the add command; which will run the appropriate function. Then, we'll pass in title, setting it equal to secret, and then we can pass in body, which will be our second command-line argument, setting that equal to the string, This is my secret:
node app.js add --title=secret --body="This is my secret"
In this command, we specified three things: the add command the title argument, which gets set to secret; and the body argument, which gets set to "This is my secret". If all goes well, we'll get the appropriate log. Let's run the command.
In the following command output, you can see Adding note secret, which is the title; and This is my secret, which is the body:

With this in place, we now have one of our methods set up and ready to go. The next thing that we'll do is convert the other commands we have—the list, read, and remove commands. Let's look into one more command, and then you'll do the other two by yourself as exercises.