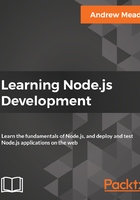
Running yargs
Now that we've installed yargs, we can move over into Atom, inside of app.js, and get started with using it. The basics of yargs, the very core of its feature set, is really simple to take advantage of. The first thing we'll do is to require it up, as we did with fs and lodash in the previous chapter. Let's make a constant and call it yargs, setting it equal to require('yargs'), as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command:', command);
console.log(process.argv);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else if (command === 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
From here, we can fetch the arguments as yargs parses them. It will take the same process.argv array that we discussed in the previous chapter, but it goes behind the scenes and parses it, giving us something that's much more useful than what Node gives us. Just above the command variable, we can make a const variable called argv, setting it equal to yargs.argv, as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
const argv = yargs.argv;
var command = process.argv[2];
console.log('Command:', command);
console.log(process.argv);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else if (command === 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
The yargs.argv module is where the yargs library stores its version of the arguments that your app ran with. Now we can print it using console.log, and this will let us take a look at the process.argv and yargs.argv variables; we can also compare them and see how yargs differs. For the command where we use console.log to print process.argv, I'll make the first argument a string called Process so that we can differentiate it in Terminal. We'll call console.log again. The first argument will be the Yargs string, and the second one will be the actual argv variable, which comes from yargs:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
const argv = yargs.argv;
var command = process.argv[2];
console.log('Command:', command);
console.log('Process', process.argv);
console.log('Yargs', argv);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else if (command === 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
Now we can run our app (refer to the preceding code block) a few different ways and see how these two console.log statements differ.
First up, we'll run at node app.js with the add command, and we can run this very basic example:
node app.js add
We already know what the process.argv array looks like from the previous chapter. The useful information is the third string inside of the array, which is 'add'. In the fourth string, Yargs gives us an object that looks very different:

As shown in the preceding code output, first we have the underscore property, then commands such as add are stored.
If I were to add another command, say add, and then I were to add a modifier, say encrypted, you would see that add would be the first argument and encrypted the second, as shown here:
node app.js add encrypted

So far, yargs really isn't shining. This isn't much more useful than what we have in the previous example. Where it really shines is when we start passing in key-value pairs, such as the title example we used in the Getting input section of Node Fundamentals - Part 1 in chapter 2. I can set my title flag equal to secrets, press enter, and this time around, we get something much more useful:
node app.js add --title=secrets
In the following code output, we have the third string that we would need to parse in order to fetch the value and the key, and in the fourth string, we actually have a title property with a value of secrets:

Also, yargs has built-in parsing for all the different ways you could specify this.
We can insert a space after title, and it will still work just as it did before; we can add quotes around secrets, or add other words, like secrets from Andrew, and it will still parses it correctly, setting the title property to the secrets from Andrew string, as shown here:
node app.js add --title "secrets from Andrew"

This is where yargs really shines! It makes the process of parsing your arguments a lot easier. This means that inside our app, we can take advantage of that parsing and call the proper functions.