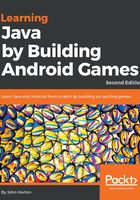
Different types of variables
It is not hard to imagine that even a simple game will have quite a few variables. In the previous section, we introduced a few, some hypothetical, some from the Sub' Hunter game. What if the game had an enemy with a width
, height
, color
, ammo
, shieldStrength
and position
variables?
Another common requirement in a computer program, including games, is the right or wrong calculation. Computer programs represent right or wrong calculations using true
or false
.
To cover these and other types of data you might want to store or manipulate, Java has types. These types are many but fall into two main categories. Primitive and reference types.
Primitive types
There are many types of variables and we can even invent our own types as well. But for now, we will look at the most used built-in Java types that we will use to make games. And to be fair, they cover most situations we are likely to run into for a while. These examples are the best way to explain types.
We have already discussed the hypothetical score
variable. This variable is, of course, a number, so we must tell the Java compiler this by giving it an appropriate type. The hypothetical playerName
will, of course, hold the characters that make up the player's name. Jumping ahead a bit, the type that holds a regular number is called int
(short for integer) and the type that holds name-like data is called String
. And if we try to store a player's name, perhaps "Jeff Minter" in an int
like score
, meant for numbers, we will certainly run into trouble, as we can see from the next screenshot:

As we can see, Java was designed to make it impossible for such errors to make it into a running program. With the compiler protecting us from ourselves, what could possibly go wrong?
Let's look through those most common types in Java, and then we will see how to start using them:
int
: Theint
type is for storing integers, whole numbers. This type uses 32 pieces (bits) of memory and can, therefore, store values with a size a little more than two billion, including negative values too.long
: As the name hints at,long
data types can be used when even larger numbers are needed. Along
type uses 64 bits of memory and 2 to the power of 63 is what we can store in this. If you want to see what that looks like, here it is 9,223,372,036,854,775,807. Perhaps, surprisingly, there are many uses forlong
variables, but the point is, if a smaller variable will do, we should use it because our program will use less memory.Note
You might be wondering when you might use numbers of this magnitude. The obvious examples would be math or science applications that do complex calculations, but another use might be for timing. When you time how long something takes, the Android
System
class uses the number of milliseconds since January 1, 1970. A millisecond is one-thousandth of a second, so there have been quite a few of them since 1970.float
: This is for floating-point numbers. That is numbers where there is precision beyond the decimal point. As the fractional part of a number takes memory space just as the whole number part does, the range of a number possible in afloat
is therefore decreased compared to non-floating-point numbers.boolean
: We will be using plenty of Booleans throughout the book. Theboolean
variable type can be eithertrue
orfalse
; nothing else. Booleans answer questions such as: Is the player alive? Are we currently debugging? Are two examples of Boolean enough?
Tip
I have kept this discussion of data types to a practical level that is useful in the context of this book. If you are interested in how a data type's value is stored and why the limits are what they are, then have a look at the Oracle Java tutorials site here: http://docs.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html. Note that you do not need any more information than we have already discussed to continue with this book.
As we just learned, each type of data that we might want to store will need a specific amount of memory. This means we must let the Java compiler know the type of the variable before we begin to use it.
Remember, these variables are known as the primitive types. They use predefined amounts of memory and so- using our warehouse storage analogy- fit into predefined sizes of the storage box.
As the "primitive" label suggests, they are not as sophisticated as reference types.
Reference variables
You might have noticed that we didn't cover the String
variable type that we previously used to introduce the concept of variables that hold alphanumeric data such as a player's name in a high score table or perhaps a multiplayer lobby.
String references
Strings are one of a special type of variable known as a reference type. They quite simply refer to a place in memory where storage of the variable begins but the reference type itself does not define a specific amount of memory used. The reason for this is straightforward.
We don't always know how much data will be needed to be stored in it until the program is executed.
We can think of Strings and other reference types as continually expanding and contracting storage boxes. So, won't one of these String
reference types bump into another variable eventually?
As we are thinking about the device's memory as a huge warehouse full of racks of labeled storage boxes, then you can think of the DVM as a super-efficient forklift truck driver that puts the distinct types of storage boxes in the most appropriate places.
And if it becomes necessary, the DVM will quickly move stuff around in a fraction of a second to avoid collisions. Also, when appropriate, Dalvik, the forklift driver, will even throw out (delete) unwanted storage boxes. This happens at the same time as constantly unloading new storage boxes of all types and placing them in the best place, for that type of variable.
Dalvik keeps reference variables in a different part of the warehouse to the primitive variables. And we will learn more details about this in Chapter 14, The Stack, the Heap, and the Garbage Collector.
So, Strings can be used to store any keyboard character. Anything from a player's initials to an entire adventure game text can be stored in a single String
.
Array references
There are a couple more reference types we will explore as well. Arrays are a way to store lots of variables of the same type, ready for quick and efficient access. We will look at arrays in Chapter 12, Handling Lots of Data with Arrays.
For now, think of an array as an aisle in our warehouse with all the variables of a certain type lined up in a precise order. Arrays are reference types, so Dalvik keeps these in the same part of the warehouse as Strings. As an example, we will use an array to store thousands of bullets in the Bullet Hell game we start in Chapter 12, Handling Lots of Data with Arrays.
Object/class references
The other reference type is the class. We have already discussed classes but not explained them properly. We will be getting familiar with classes in Chapter 8, Object-Oriented Programming.
Now we know that each type of data that we might want to store will need an amount of memory. Hence, we must let the Java compiler know the type of the variable before we begin to use it.