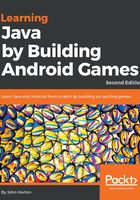
Java Variables
I have already mentioned that variables are our data. Data is simply, values. We can think of a variable as a named storage box. We choose a name, perhaps livesRemaining
. These names are kind of like our programmer's window into the memory of the user's Android device.
Variables are values in computer memory ready to be used or altered when necessary by using the name they were given.
Computer memory has a highly complex system of addressing, which fortunately we do not need to interact with because it is handled by the operating system. Java variables allow us to devise our own convenient names for all the data we need our program to work with.
The DVM (Dalvik Virtual Machine) will handle all the technicalities of interacting with the operating system, and the operating system will, in turn, interact with the physical memory, the RAM microchips.
So, we can think of our Android device's memory as a huge warehouse just waiting for us to add our variables. When we assign names to our variables, they are stored in the warehouse, ready for when we need them. When we use our variable's name, the device knows exactly what we are referring to. We can then tell it to do things such as: get livesRemaining
and multiply it by bonusAmount
, set it to zero, and so on.
In a typical game, we might have a variable named score
, to hold the player's current score. We could add to it when the player shoots another alien and subtract from it if the player shoots a civilian.
Some situations that might arise are:
- Player completes a level so add
levelBonus
toplayerScore
- Player gets a new high score so assign the value of
score
tohighScore
- Player starts a new game so reset
score
to zero
These are realistic example names for variables and if you don't use any of the characters or keywords that Java restricts, you can call your variables whatever you like.
To help your game project succeed it is useful to adopt a naming convention so that your variable names will be consistent. In this book, we will use a loose convention of variable names starting with a lowercase letter. When there is more than one word in the variable's name, the second word will begin with an uppercase letter.
Note
We call this camel casing. For example, camelCasing
.
Here are some variable examples of the Sub' Hunter game that we will soon use:
shotsTaken
subHorizontalPosition
debugging
The earlier examples also imply something.
- The variable
shotsTaken
is probably going to be a whole number. subHorizontalPosition
sounds like a coordinate, perhaps a decimal fraction (floating point number)- And,
debugging
sounds more like a question or statement than a value.
We will see shortly that indeed there are distinct types of variable.
Note
As we move on to object-oriented programming we will use extensions to our naming convention for special types of variables.
Before we look at some more Java code with some variables, we need to first look at the types of variables we can create and use.