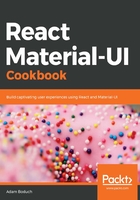
There's more...
You might want to separate the rendering of list items into its own component. This way, you can use the list items in other places. For example, you might want to use the same rendering logic to render a list of buttons elsewhere in your app. Here's an example of how you can extract the ListItems component into its own component:
const ListItems = ({ items, onClick }) =>
items
.filter(({ hidden }) => !hidden)
.map(({ label, disabled, Icon }, i) => (
<ListItem
button
key={i}
disabled={disabled}
onClick={onClick(label)}
>
<ListItemIcon>
<Icon />
</ListItemIcon>
<ListItemText>{label}</ListItemText>
</ListItem>
));
The ListItems component will return an array of ListItem components. It takes the items state to render as an array property. It also takes an onClick() function property. This is a higher-order function that takes the label component to display as an argument and returns a new function that will update the content when the item is clicked on.
Here's what the new JSX markup looks like, updated to use the new ListItems component:
<Grid container justify="space-between">
<Grid item>
<Typography>{content}</Typography>
</Grid>
<Grid item>
<Drawer open={open} onClose={() => setOpen(false)}>
<List>
<ListItems items={items} onClick={onClick} />
</List>
</Drawer>
</Grid>
<Grid item>
<Button onClick={() => setOpen(!open)}>
{open ? 'Hide' : 'Show'} Drawer
</Button>
</Grid>
</Grid>
There is no more list item rendering code in this component. Instead, ListItems is rendered as the child of List. You pass it the items to render and the onClick() handler. You now have a generic ListItems component that can be used anywhere that you show lists in your app. It will consistently handle the Icon, disabled, and display logic wherever it is used.