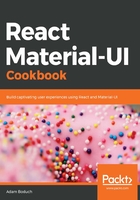
How it works...
Let's start by looking at the state of your component:
const [open, setOpen] = useState(false);
const [content, setContent] = useState('Home');
const [items] = useState([
{ label: 'Home', Icon: HomeIcon },
{ label: 'Page 2', Icon: WebIcon },
{ label: 'Page 3', Icon: WebIcon, disabled: true },
{ label: 'Page 4', Icon: WebIcon },
{ label: 'Page 5', Icon: WebIcon, hidden: true }
]);
The open state controls the visibility of the Drawer component, and the content state is the text that's displayed on the screen depending on which drawer item is clicked on. The items state is an array of objects that is used to render the drawer items. Every object has a label property and an Icon property that are used to render the item text and icon respectively.
The disabled property is used to render the item as disabled; for example, Page 3 is marked as disabled by setting this property to true:

This could be due to permission restrictions for the user on this particular page, or some other reason. Because this is controlled through the component state instead of rendered statically, you could update the disabled state for any menu item at any time using any mechanism that you like, such as an API call. The hidden property uses the same principle, except when this value is true, the item isn't rendered at all. In this example, Page 5 isn't rendered because it's marked as hidden.
Next, let's look at how the List items are rendered based on the items state, as follows:
<List>
{items
.filter(({ hidden }) => !hidden)
.map(({ label, disabled, Icon }, i) => (
<ListItem
button
key={i}
disabled={disabled}
onClick={onClick(label)}
>
<ListItemIcon>
<Icon />
</ListItemIcon>
<ListItemText>{label}</ListItemText>
</ListItem>
))}
</List>
First, the items array is filtered to remove hidden items. Then, map() is used to render each ListItem component. The disabled property is passed to ListItem and it will be visibly disabled when rendered. The Icon component also comes from the list item state. The onClick() event handler hides the drawer and updates the content label.