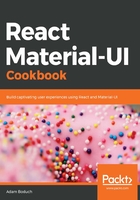
How it works...
Let's start by taking a look at the Routes component that define the pages in your app, as follows:
const WithNavigation = withStyles(styles)(({ classes }) => (
<div className={classes.root}>
<Route
exact
path="/"
render={() => (
<Fragment>
<MyToolbar title="Home" />
<Typography>Home</Typography>
</Fragment>
)}
/>
<Route
exact
path="/page2"
render={() => (
<Fragment>
<MyToolbar title="Page 2" />
<Typography>Page 2</Typography>
</Fragment>
)}
/>
<Route
exact
path="/page3"
render={() => (
<Fragment>
<MyToolbar title="Page 3" />
<Typography>Page 3</Typography>
</Fragment>
)}
/>
</div>
));
Each Route component (from the react-router package) corresponds to a page in your app. They have a path property that matches the path in the browser address bar. When there's a match, this Routes component' content is rendered. For example, when the path is /page3, the content for the Route component where path="/page3" is rendered.
Each Route component also defines a render() function. This is called when its path is matched and the returned content is rendered. The Routes component in your app each render MyToolbar with a different value for the title prop.
Next, let's take a look at the menu items that make up the MenuItems default property value, as follows:
static defaultProps = {
MenuItems: () => (
<Fragment>
<MenuItem component={Link} to="/">
Home
</MenuItem>
<MenuItem component={Link} to="/page2">
Page 2
</MenuItem>
<MenuItem component={Link} to="/page3">
Page 3
</MenuItem>
</Fragment>
),
RightButton: () => <Button color="inherit">Login</Button>
};
Each of these MenuItems properties is a link that points to each of the Routes component declared by your app. The MenuItem component accepts a component property that is used to render the link. In this example, you're passing it the Link component from the react-router-dom package. The MenuItem component will forward any additional properties to the Link component, which means that you can can pass the to property to the MenuItem component and it's as though you're passing it to the Link component.