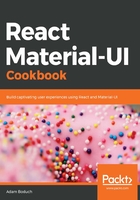
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you're building an app that has three pages. On each page, you want to render an App Bar with the title prop of the page. Furthermore, the menu in the App Bar should contain links to the three pages. Here's how to do it:
import React, { Fragment, Component } from 'react';
import {
BrowserRouter as Router,
Route,
Link
} from 'react-router-dom';
import { withStyles } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import IconButton from '@material-ui/core/IconButton';
import MenuIcon from '@material-ui/icons/Menu';
import Menu from '@material-ui/core/Menu';
import MenuItem from '@material-ui/core/MenuItem';
const styles = theme => ({
root: {
flexGrow: 1
},
flex: {
flex: 1
},
menuButton: {
marginLeft: -12,
marginRight: 20
},
toolbarMargin: theme.mixins.toolbar
});
const MyToolbar = withStyles(styles)(
class extends Component {
static defaultProps = {
MenuItems: () => (
<Fragment>
<MenuItem component={Link} to="/">
Home
</MenuItem>
<MenuItem component={Link} to="/page2">
Page 2
</MenuItem>
<MenuItem component={Link} to="/page3">
Page 3
</MenuItem>
</Fragment>
),
RightButton: () => <Button color="inherit">Login</Button>
};
state = { anchor: null };
closeMenu = () => this.setState({ anchor: null });
render() {
const { classes, title, MenuItems, RightButton } = this.props;
return (
<Fragment>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
onClick={e =>
this.setState({ anchor: e.currentTarget })
}
>
<MenuIcon />
</IconButton>
<Menu
anchorEl={this.state.anchor}
open={Boolean(this.state.anchor)}
onClose={this.closeMenu}
>
<MenuItems />
</Menu>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
{title}
</Typography>
<RightButton />
</Toolbar>
</AppBar>
<div className={classes.toolbarMargin} />
</Fragment>
);
}
}
);
const WithNavigation = withStyles(styles)(({ classes }) => (
<div className={classes.root}>
<Route
exact
path="/"
render={() => (
<Fragment>
<MyToolbar title="Home" />
<Typography>Home</Typography>
</Fragment>
)}
/>
<Route
exact
path="/page2"
render={() => (
<Fragment>
<MyToolbar title="Page 2" />
<Typography>Page 2</Typography>
</Fragment>
)}
/>
<Route
exact
path="/page3"
render={() => (
<Fragment>
<MyToolbar title="Page 3" />
<Typography>Page 3</Typography>
</Fragment>
)}
/>
</div>
));
export default WithNavigation;
Here's what you'll see when you first load the app:

Here's what the menu in the App Bar looks like when it's opened:

Try clicking on Page 2; here's what you should see:

The title of the App Bar has changed to reflect the title of the page, and the content of the page has also changed.