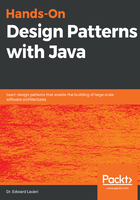
InterpreterDriver class
The InterpreterDriver class is the class that drives the application and therefore contains the main() method. There are two class variables, originatingContent and theExpression; both are initially set to null.
In addition to the constructor method, there is also an interpret() method that does the following:
- Uses the Scanner class
- Instantiates a new Expression instance
- Calls the interpret() method on the new Expression instance
The InterpreterDriver class is provided next:
import java.util.Scanner;
public class InterpreterDriver {
// class variables
public Conversion originatingContent = null;
public Expression theExpression = null;
public InterpreterDriver(Conversion content) {
originatingContent = content;
}
public void interpret(String tString) {
Scanner in = new Scanner(System.in);
theExpression = new MapIntToCharacters(tString);
theExpression.interpret(originatingContent);
}
Execution of the InterpreterDriver main() method starts by printing the output header, CODE INTERPRETER, and prompts users for their input. Next, the Scanner class is used to obtain user input via their keyboard. That input is used to create a new Conversion instance. That instance is used to instantiate a new InterpreterDriver object. Finally, the interpret() method is called on that InterpreterDriver object:
public static void main(String[] args) {
System.out.println("\n\nCODE INTERPRETER\n");
System.out.print("Enter your code: ");
Scanner in = new Scanner(System.in);
String userInput = in.nextLine();
System.out.println("Your code: " + userInput);
Conversion conversion = new Conversion(userInput);
InterpreterDriver userCode = new InterpreterDriver(conversion);
userCode.interpret(userInput);
System.out.println("\n\n");
}
}
The program's output is provided here:

This section featured the source code, demonstrating the interpreter design pattern.