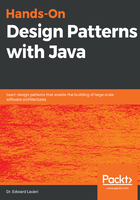
The Conversion class
The Conversion class applies when there is conversion from numbers to letters (int to char). The class has a single String class variable and a constructor method that is passed to the user's input. The class has one additional method, the convertToCharacters() method. That method converts the user's input into a character array and then processes that array, one character at a time. The processing is implemented with a simple switch statement. Each numerical value, from 0 through 9, has a corresponding character. The characters are printed one at a time until the entire character array has been processed.
The first section of our Conversion class follows:
public class Conversion {
// class variable
public String userInput;
// constructor
public Conversion(String userInput) {
this.userInput = userInput;
}
public void convertToCharacters(String userInput) {
this.userInput = userInput;
System.out.print("Decrypted Message: ");
char answer[] = userInput.toCharArray();
The remaining code from the Conversion class is provided here:
for (int i=0; i < answer.length; i++) {
switch (answer[i]) {
case '0':
System.out.print("A");
break;
case '1':
System.out.print("E");
break;
case '2':
System.out.print("I");
break;
case '3':
System.out.print("Y");
break;
case '4':
System.out.print("O");
break;
case '5':
System.out.print("L");
break;
case '6':
System.out.print("R");
break;
case '7':
System.out.print("T");
break;
case '8':
System.out.print("C");
break;
case '9':
System.out.print("S");
break;
}
}
}
}
The preceding code implements a 10-character mapping of integers. A complete implementation would account for the entire alphabet or, depending on the language, alphabets.