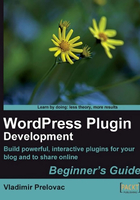
Time for action – Apply CSS to the popup
In this example, we are going to style our pop up using the CSS. The contents of the CSS will be located in the external file for easy editing. We will use another WordPress action to load this stylesheet from the <head>
tag of the document.
- Create a new file named
wp-live-blogroll.css
. - Add this code to style the
lb_popup
element:#lb_popup { color:#3366FF; width:250px; border:2px solid #0088CC; background:#fdfdfd; padding:4px 4px; display:none; position:absolute; }
- Edit our JavaScript
wp-live-blogroll.js
to position the pop up at mouse coordinates:// create a new div and display a tip inside $(this).append('<div id="lb_popup">' + this.tip + '</div>'); // get coordinates var mouseX = e.pageX || (e.clientX ? e.clientX + document.body.scrollLeft: 0); var mouseY = e.pageY || (e.clientY ? e.clientY + document.body.scrollTop: 0); // move the top left corner to the left and down mouseX -= 260; mouseY += 5; // position our div $('#lb_popup').css({ left: mouseX + "px", top: mouseY + "px" }); // show it using a fadeIn function $('#lb_popup').fadeIn(300);
- Finally, load the CSS style by using the
wp_head
action. Edit thewp-live-blogroll.php
file and add this code to the end:add_action('wp_head', 'WPLiveRoll_HeadAction' ); function WPLiveRoll_HeadAction() { global $wp_live_blogroll_plugin_url; echo '<link rel="stylesheet" href="'.$wp_live_blogroll_plugin_url.'/wp-live-blogroll.css" type="text/css" />'; }
- Be sure to upload all new files to the server. Once you reload the page you should get a newly created pop-up window just below the mouse pointer, that looks like this:
What just happened?
We created a pop-up window by applying CSS styling to it and placed it according to the current mouse position.
Our stylesheet is loaded using the wp_head
(for admin pages wp_admin_head) action executed inside the <head>
tag of the page:
add_action('wp_head', 'WPLiveRoll_HeadAction' ); function WPLiveRoll_HeadAction() { global $wp_live_blogroll_plugin_url; echo '<link rel="stylesheet" href="'.$wp_live_blogroll_plugin_url.'/wp-live-blogroll.css"type="text/css" />'; }
In the CSS file we use '#
' to reference our identifier (<div id='lb_popup'>
). If we were referencing a class (possibly multiple elements) we would use a '.' instead):
#lb_popup { color:#3366FF; width:250px; border:2px solid #0088CC; background:#fdfdfd; padding:4px 4px; display:none; position:absolute; }
The style specifies several attributes including the width, font color and background color. We set the position to absolute as we will tell it exactly where to appear with the JavaScript. We also want to show the pop up using jQuery fade-in effect, so we set display to none as a default value.
The next snippet of code calculates the position of the mouse on our page:
// get coordinates var mouseX = e.pageX || (e.clientX ? e.clientX + document.body.scrollLeft: 0); var mouseY = e.pageY || (e.clientY ? e.clientY + document.body.scrollTop: 0);
We then offset the coordinates by a predefined amount to get the final desired position:
// move the top left corner to the left and down mouseX -= 260; mouseY += 5;
jQuery offers various functions for displaying hidden elements such as show, slide, animate and fadeIn.
In our example, we use the fadeIn
effect with the optional parameter of fade-in speed in milliseconds:
// show it using a fadeIn function $('#lb_popup).fadeIn(300);
Having solved the pop up, it's finally time to use Ajax. Lots of people still avoid using Ajax as the technology is still new. But we will show how to use it in a very simple manner.