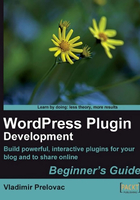
Time for action – Creating a hover event with jQuery
We will create an external file to store our JavaScript and use it to display a message directly in the blogroll, when the user hovers the mouse over one of the links.
- Create a new file called
wp-live-blogroll.js.
- Initialize jQuery when our page loads:
// setup everything when document is ready jQuery(document).ready(function($) {
- Assign a hover event to our links.
// connect to hover event of <a> in .livelinks $('.livelinks a').hover(function(e) {
- Insert a new element (
<div>
) on the mouse hover event. It will display a simple message for now (Recent posts will be displayed here) :// set the text we want to display this.tip="Recent posts from " + this.href + " will be displayed here..."; // create a new div and display a tip inside $(this).append('<div id="lb_popup">' + this.tip + '</div>'); },
- And remove it when the user moves the mouse:
// when the mouse hovers out function() { // remove it $(this).children().remove(); }); });
- Using jQuery we have just assigned hover event to class 'livelinks'.
That means that blogroll needs to be embedded within this class. Open up our plugin file
wp-live-blogroll.php
and add the following filter function to the end. It will embed our blogroll into thelivelinks
class.add_filter('wp_list_bookmarks', WPLiveRoll_ListBookmarksFilter); function WPLiveRoll_ListBookmarksFilter($content) { return '<span class="livelinks">'.$content.'</span>'; }
- Finally, we want to have WordPress load our script when the page loads.
We use the
wp_print_scripts
action to accomplish that and thewp_enqueue_script
function to include our JavaScript:add_action('wp_print_scripts', 'WPLiveRoll_ScriptsAction'); function WPLiveRoll_ScriptsAction() { global $wp_live_blogroll_plugin_url; if (!is_admin()) { wp_enqueue_script('jquery'); wp_enqueue_script('wp_live_roll_script', $wp_live_blogroll_plugin_url.'/wp-live-blogroll.js', array('jquery')); } }
- We do not want to use the get_bookmarks filter anymore. We will simply comment out the filter assignment:
add_filter('get_bookmarks', WPLiveRoll_GetBookmarksFilter);
- Update the plugin on the server. Try to hover the mouse over one of the links and you should get our message.
What just happened?
Our script is loaded in the <head>
section of the page with the wp_print_scripts
action, which is used to print out scripts our plugin will use.
add_action('wp_print_scripts', 'WPLiveRoll_ScriptsAction');
To actually add the scripts, we use the wp_enqueue_script()
WordPress function, which will be covered in detail later. This function allows us to add preinstalled scripts like jQuery, or our custom scripts:
function WPLiveRoll_ScriptsAction() { global $wp_live_blogroll_plugin_url; if (!is_admin()) { wp_enqueue_script('jquery'); wp_enqueue_script('wp_live_roll_script', $wp_live_blogroll_plugin_url.'/wp-live-blogroll.js', array('jquery')); } }
Next, Blogroll is embedded in the livelinks
class, so our jQuery code can properly assign the hover event. We used the wp_list_bookmarks
hook, which executes just before the blogroll shows on the page.
add_filter('wp_list_bookmarks', WPLiveRoll_ListBookmarksFilter);
The filter function receives the full HTML code of the prepared blogroll, so we only need to embed its content into our livelinks
class and return it:
function WPLiveRoll_ListBookmarksFilter($content) { return '<span class="livelinks">'.$content.'</span>'; }
To work with jQuery when the page is ready, we need to initialize it at the beginning of the JavaScript code. jQuery provides the ready
method which executes when the page is loaded (more accurately when the page code is loaded; the images may still be loading):
// set up everything when document is ready jQuery(document).ready(function($) {
Next, we set up a hover jQuery event for links inside the livelinks
class using a jQuery selector (more on that later).
// connect to hover event of <a> in .livelinks $('.livelinks a').hover(function(e) {
Now, we want to add a text and a <div>
to display it in. We used the internal variable this
, which represents the object that triggered the hover event (which will be one of the links in the Blogroll).
To store the temporary value of the text, we will create a new variable tip
and use the URL of the link found in the link's this.href
property:
// set the text we want to display this.tip="Recent posts from " + this.href + " will be displayed here...";
We then dynamically append code to our link by using the jQuery append()
method:
// create a new div and display a tip inside $(this).append('<div id="lb_popup">' + this.tip + '</div>');
The hover event allows us to declare the function for handling the hover out event and we use it to remove the <div>
when the user moves the mouse away from the link.
To do that, we can use the children()
method, which references elements under our link, in this case, the appended div:
// when the mouse hovers out function() { // remove it $(this).children().remove(); } });
JavaScript and WordPress
WordPress provides a way to add external scripts in an elegant way, by using the wp_enqueue_script
function.
The wp_enqueue_script
function is usually used in conjunction with the wp_print_scripts
action and accepts up to four parameters: handle
, src
, dependencies
, and version
.
handle
: This is the name of the script, it should be a lowercase string.src
(Optional): This is the path to the script from the root directory of WordPress, example:/wp-includes/js/scriptaculous/scriptaculous.js
. This parameter is required only when WordPress does not already know about this script. It defaults to false.dependencies
(Optional): This is an array of the handles of any scripts that this script depends on; which means, that the scripts that must be loaded before this script. Set it to false if there are no dependencies. This parameter is required only when WordPress does not already know about this script. It defaults to false.version
(Optional): This is the string specifying a script version number, if it has one. It defaults to false. This parameter is used to ensure that the correct version is sent to the client regardless of caching, and should therefore be included if a version number is available and makes sense for the script.
In our example, we declared that we are using jQuery and then our own script by specifying the full path to it:
wp_enqueue_script('jquery');
wp_enqueue_script('wp_live_roll_script', $wp_live_blogroll_plugin_url.'/wp-live-blogroll.js',array('jquery'));
As mentioned before, WordPress comes preinstalled with a variety of scripts. To use them, you need to specify the handle to wp_enqueue_script()
, as we did with jQuery. Some of the popular scripts included are dbx (Docking Boxes), colorpicker, wp_tiny_mce
(WordPress Tiny MCE), autosave, scriptaculous, prototype, thickbox and others.
Note
Quick reference
wp_enqueue_script()
: This is used to queue an external script used by our plugin. Additional information is available at http://codex.wordpress.org/Function_Reference/wp_enqueue_script
bloginfo()
: This is the function used to retrieve relevant blog information like the homepage URL and print it out. get_bloginfo
also does the same thing; it only returns the information instead of printing it. More information is available at: http://codex.wordpress.org/Template_Tags/bloginfo
wp_print_scripts
: Filter which runs inside the <head>
tag of the document, usually used to declare scripts
We have initialized jQuery with this code:
// set up everything when document is ready jQuery(document).ready(function($) {
Notice the '$
' parameter to the function? All jQuery variables and functions are constrained within the jQuery namespace. You can usually access them using jQuery()
and also $()
. The only problem is that sometimes other libraries also use $
as their namespace reference, which leads to conflicts.
The most elegant way to avoid this is to declare the ready function as we did, which allows us to use the shorter $
reference inside the ready()
function while avoiding conflict with other libraries.
One of the advantages of jQuery is the ability to access every single element in our document with ease. Let's try a few examples:
$("h1")
: gets all the<h1>
tags$("#sidebar")
: gets the element withid="sidebar"
$(".navigation")
: gets all the elements withclass="navigation"
$("div.post #image")
: gets the element withid="image"
nested in the<div class="post">
$("#sidebar ul li")
: gets all the<li>
elements nested in all<ul>
, under the element withid="sidebar"
More control is given with the commands such as first, last, contains, visible, for example:
$("li:first")
: selects the first instance$("li:contains(blog)")
: selects all the elements in<li>
that contain the text "blog"$("li:gt(n)")
: selects all the elements in<li>
with an index greater than(n)
Responding to user events is a breeze in jQuery. There are custom defined events that we can use such as: click
, mouseover
, hover
, keydown
, focus
, and so on.
This code will add an alert pop-up whenever you click on any link on the page:
$(document).ready(function() { $("a").click(function() { alert("Thanks for visiting!"); }); });
jQuery allows us to modify the document by adding classes to the elements (addclass
) or binding events to them easily.
$(document).ready(function() { $('#button').bind('click', function(e) { alert('The mouse is now at ' + e.pageX + ', ' + e.pageY); }); });
jQuery also supports plugins, and there are literally hundreds of them which allows for almost any kind of effect or functionality that you may imagine.
Tip
A good resource to start browsing for jQuery examples and documentation is the official web site at http://www.jquery.com and Learning jQuery web site at http://www.learningjquery.com.
Creating the pop-up with CSS
Let's move on with our plugin and create a pop-up window for our message. To do this, we will include CSS code and make a couple of changes to the plugin.