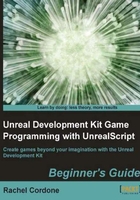
Time for action – Creating a custom GameInfo and PlayerController
Creating a custom GameInfo
class is simple enough; it's just knowing where to let the game know that you want to run it. First up, let's create the class.
- Create a new file in our
AwesomeGame/Classes
folder calledAwesomeGame.uc
. Type the following code into it:class AwesomeGame extends UTDeathmatch; defaultproperties { }
UTDeathmatch
is a good place to start for a customGameInfo
, even for games that don't involve killing anyone, or for single player games.UTDeathmatch
and its parent classes have a lot of functionality in common with those types of games including the player spawn behavior. - Now let's create a custom
PlayerController
. Create a new file in ourAwesomeGame/Classes
folder calledAwesomePlayerController.uc
. Type the following code into it:class AwesomePlayerController extends UTPlayerController; simulated function PostBeginPlay() { super.PostBeginPlay(); `log("AwesomePlayerController spawned!"); } defaultproperties { }
We are almost done with the code part. One other thing that a
GameInfo
class does is control what type ofPlayerController
is spawned. If you wanted to have a class-based game you could do it by creating more than one customPlayerController
class and using your customGameInfo
to spawn the one the player selects. - For
AwesomeGame
we're only using one type ofPlayerController
, so let's set that in the default properties.class AwesomeGame extends UTDeathmatch; defaultproperties { PlayerControllerClass=class'AwesomeGame.AwesomePlayerController' }
Remember that the first part of the property,
AwesomeGame
, depends on what you named your folder underDevelopment\Src
.PlayerControllerClass
is a variable declared inGameInfo
and inherited by all subclasses ofGameInfo
including ourAwesomeGame
. - Compile the code.
- The map we've been using up until now has been fine for our purposes, but we're going to need something with a little more room to experiment from now on. If you're familiar with the editor then create a simple flat map with a player start at the center and lights so we can see. If you'd rather just get to the programming, place the file called
AwesomeTestMap.udk
included with the book into theUDKGame/Content/Maps/AwesomeGame
folder that we created in Chapter 1. - Time to run our game! Since the editor can't be open while we compile, and starting up the editor takes a bit of time, I prefer to use batch files to run test maps. It saves a lot of time and they're easy to set up. Create a text file anywhere that's convenient for you and call it
Awesome Test Map.txt
. Write the following in it:C:\UDK\UDK-AwesomeGame\Binaries\Win32\UDK.exe AwesomeTestMap?GoalScore=0?TimeLimit=0?Game=AwesomeGame.AwesomeGame -log
Make sure all the punctuation is correct, there are only two spaces after
UDK.exe
and before-log
. If you've installed the UDK in a different location or under a different name, be sure to write down your correct path toUDK.exe
. This file is also included with the book if you're unsure of the format. Once again remember that the first part ofAwesomeGame.AwesomeGame
refers to the name of the.u
file you have compiled. - Save the text file, and then rename it to change the extension from
.txt
to.bat
. - Double-click on the file to run the test map. You'll notice that the DOS window that pops up looks really familiar. Adding
-log
to the end of our batch file makes it so that we can see the log being written as it happens. If we look carefully at it, or shut down the game and openLaunch.log
(notLaunch2.log
, we're not running the editor now), we can see our log show up:[0005.67] ScriptLog: AwesomePlayerController spawned!
Awesome!
What just happened?
Now we know that our code is working correctly. The batch file is telling the game to use our custom GameInfo
class, which is telling the game to use our custom PlayerController
. When setting up a new UDK project these are usually the first two classes that get created, so now we have a good starting point for creating a custom game. So what can we do now?