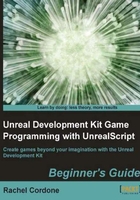
Time for action – Using switches
- Let's take a look at an example of a Switch statement.
var int Int1; function PostBeginPlay() { Int1 = 2; switch(Int1) { case 1: 'log("Int1 == 1"); case 2: 'log("Int1 == 2"); case 3: 'log("Int1 == 3"); default: 'log("Int1 isn't any of those!"); } }
- Running the code, the log looks like this:
[0007.97] ScriptLog: Int1 == 2 [0007.97] ScriptLog: Int1 == 3 [0007.97] ScriptLog: Int1 isn't any of those!
What just happened?
Why did the other lines log? Unlike if/else statements, switches will continue executing the next steps after the condition is met. Sometimes we'll want it to do that, but if not we can use the break statement here too.
var int Int1; function PostBeginPlay() { Int1 = 2; switch(Int1) { case 1: 'log("Int1 == 1"); break; case 2: 'log("Int1 == 2"); break; case 3: 'log("Int1 == 3"); break; default: 'log("Int1 isn't any of those!"); } }
The log file for this would have our desired behavior.
[0007.69] ScriptLog: Int1 == 2
Return
Return simply exits out of a function. This is most commonly combined with other flow control statements like if/else. Take the following code:
var int Int1; function PostBeginPlay() { if(Int1 == 5) { 'log("Int1 equals 5"); return; } 'log("This will not log"); } defaultproperties { Int1=5 }
We can see what happens in the log:
[0007.83] ScriptLog: Int1 equals 5
Once the code reaches the return
statement, it stops running any more code in that function.
Goto
Goto jumps to a specific place in a function. If we had the following code:
function PostBeginPlay() { 'log("PostBeginPlay"); goto EndOfFunction; 'log("This will not log"); EndOfFunction: 'log("This will log."); }
The log would look like this:
[0007.55] ScriptLog: PostBeginPlay [0007.55] ScriptLog: This will log.
Like Return, Goto isn't really useful on its own and is more commonly combined with other flow control statements.
Pop quiz – Variable madness!
- What is the difference between an integer and a float?
- What type of variable is a vector?
- How do we make a variable changeable in the editor?
a. Add it to a config file.
b. Add parentheses after var.
c. Add it to the default properties.
- How would we write "If there's no water we will be thirsty" using logical operators?