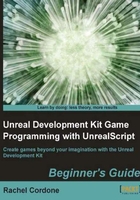
Flow control
We learned about comparisons and logical operators earlier. Now what do we do if we want different things to happen depending on the results of those comparisons? Flow control helps us do exactly that. Let's learn how we can specify what happens under different circumstances.
If else
If/else is the basic flow control statement. Let's look at this sentence:
If it's raining I'll take an umbrella.
Using an if statement, that sentence would be written like this:
if(bRaining) { bUmbrella = true; }
We could also add an else statement to it:
If it's raining I'll take an umbrella, otherwise I'll wear short sleeves.
That would be written like this:
if(bRaining) { bUmbrella = true; } else { bShortSleeves = true; }
We can also use Else If for other conditions.
If it's raining I'll take an umbrella, or if it's cold I'll wear a coat, otherwise I'll wear short sleeves.
We could write that like this:
if(bRaining) { bUmbrella = true; } else if(Temperature < ComfortableTemperature) { bCoat = true; } else { bShortSleeves = true; }
The important thing to remember about else/if is, that only one of these conditions will run. If it's raining and cold, only the bRaining
section of the code will run, not bRaining
and Temperature < ComfortableTemperature
.