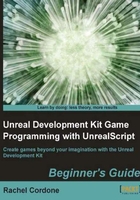
Time for action – Using structs
Going back to our basket of kittens example, what if there were other things in the basket besides kittens? How would we represent them?
- Let's create a struct at the top of our AwesomeActor class and put a few things in it.
struct Basket { var string BasketColor; var int NumberOfKittens, BallsOfString; var float BasketSize; };
Now we have two types of items in the basket as well as some variables to describe the basket itself.
- Now we need a variable of that struct so we can use it:
var Basket MyBasket;
- Now we can change the values in our
PostBeginPlay
function.function PostBeginPlay() { MyBasket.NumberOfKittens = 4; MyBasket.BasketColor = "Yellow"; MyBasket.BasketSize = 12.0; MyBasket.BallsOfString = 2;}
That seems easy enough to handle. Let's try something a bit more complex.
- I heard you like structs, so we'll Inception-ize it by adding a struct inside a struct.
struct SmallBox { var int Chocolates; var int Cookies; };
- Now let's put a box inside our basket struct.
struct Basket { var SmallBox TheBox; var string BasketColor; var int NumberOfKittens, BallsOfString; var float BasketSize; };
Now our class should look like this:
class AwesomeActor extends Actor placeable; struct SmallBox { var int Chocolates; var int Cookies; }; struct Basket { var SmallBox TheBox; var string BasketColor; var int NumberOfKittens, BallsOfString; var float BasketSize; }; var Basket MyBasket; function PostBeginPlay() { MyBasket.NumberOfKittens = 4; MyBasket.BasketColor = "Yellow"; MyBasket.BasketSize = 12.0; MyBasket.BallsOfString = 2; } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' HiddenGame=True End Object Components.Add(Sprite) }
- Now we're getting somewhere. How would we access the struct inside a struct? Using the same method as before, we would access the box like this:
MyBasket.TheBox
So accessing variables inside the box struct would look like this:
MyBasket.TheBox.Chocolates = 2;
- Easy enough. It would work the same way with arrays. Let's say we added a static array of fortune cookie messages to our basket. Our new struct would look something like this:
struct Basket { var string Fortunes[4]; var SmallBox TheBox; var string BasketColor; var int NumberOfKittens, BallsOfString; var float BasketSize; };
- To assign a value to one of them, we would use the same method as before:
MyBasket.Fortunes[2] = "Now you're programming!";
What just happened?
Structs are a powerful tool in UnrealScript, and they're used extensively in the source code. A lot of the code you'll make will involve structs. As an example, think about the one thing that every actor in the game has: A location. How is an actor's location stored? A variable appropriately called Location, which is declared as a 3D position using a Vector variable. If we look in Actor.uc
, we can see how a Vector is defined:
struct immutable Vector { var() float X, Y, Z; };
This leads nicely into our next topic...