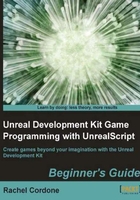
Time for action – Using arrays
- Change our variable declaration line to this:
var int Baskets[4];
This will create an array of four baskets. That's easy enough, but how do we change their values?
- In our
PostBeginPlay
function, add these lines:Baskets[0] = 2; Baskets[1] = 13; Baskets[2] = 4; Baskets[3] = 1;
One important thing to remember about arrays is that they start at 0. Even though we have 4 elements in our array, since it starts at 0 it only goes up to 3. If we tried to add a line like this to our function:
Baskets[4] = 7;
We would get an error.
- Let's go ahead and add the line to see what happens. It will compile just fine, but when we test it in the game we will see the error in the log file:
[0007.53] ScriptWarning: Accessed array 'AwesomeActor_0.Baskets' out of bounds (4/4) AwesomeActor UEDPCAwesomeMap.TheWorld:PersistentLevel.AwesomeActor_0 Function AwesomeGame.AwesomeActor:PostBeginPlay:0046
The out of bounds error lets us know that we tried to access an element of the array that doesn't exist. It takes a bit of getting used to; just remember that the first element of our array will always be 0.
- Let's take that line out of our function, and change the log line to look like this:
'log("Baskets:" @ Baskets[0] @ Baskets[1] @ Baskets[2] @ Baskets[3]);
Now our
PostBeginPlay
function should look like this:function PostBeginPlay() { Baskets[0] = 2; Baskets[1] = 13; Baskets[2] = 4; Baskets[3] = 1; 'log("Baskets:" @ Baskets[0] @ Baskets[1] @ Baskets[2] @ Baskets[3]); }
- Let's compile and test. In our log file we should see this:
[0007.53] ScriptLog: Baskets: 2 13 4 1
Success!
- Now for something a bit more complicated. We can also access the elements of our array with an int. Let's see if we can do that. Let's declare an int:
var int TestNumber;
- Then set it at the beginning of
PostBeginPlay
:TestNumber = 2;
- Now let's access the array with it and log the result.
'log("Test Basket:" @ Baskets[TestNumber]);
So now our class should look like this:
class AwesomeActor extends Actor placeable; var int Baskets[4]; var int TestNumber; function PostBeginPlay() { TestNumber = 2; Baskets[0] = 2; Baskets[1] = 13; Baskets[2] = 4; Baskets[3] = 1; 'log("Test Basket:" @ Baskets[TestNumber]); } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' HiddenGame=True End Object Components.Add(Sprite) }
- Now compile and test, then look at the log.
[0007.99] ScriptLog: Test Basket: 4
We can see that it logged the value of Basket[2], which is 4.
What just happened?
We can start to see how powerful arrays can be. We can make an array out of any variable type except for booleans, but there are ways around that. If we used an array of ints and used 0 for false and 1 for true, it could act as a boolean array.
Now we know how to make an array with a specific number of elements, but what if we don't know the number of baskets, or want to change how many baskets there are while the game is running? In that case we'll want to use a dynamic array.