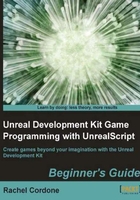
Time for action – Using strings
Well, by now we know the drill, so let's declare a string!
- Change our float declaration to this:
var string DeathMessage;
- By default, strings are empty. We can see this... or not see this, rather, by changing our
PostBeginPlay
function:function PostBeginPlay() { 'log("Death message:" @ DeathMessage); }
- Compile and test to see that nothing shows up:
[0007.74] ScriptLog: Death message:
Well that doesn't help much. Let's change that.
- Add this line to our PostBeginPlay function:
DeathMessage = "Tom Stewart killed me!";
Now it looks like this:
function PostBeginPlay() { DeathMessage = "Tom Stewart killed me!"; 'log("Death message:" @ DeathMessage); }
- Compile and run the code, and check the log.
[0007.67] ScriptLog: Death message: Tom Stewart killed me!
What just happened?
There's not much to strings either, and they're not used nearly as much as other types of variables. They're mostly used for things that need to be made readable to the player like character or weapon names, or messages on the HUD. A few are used for other things like telling the game which level to load.
Enums
Enumerations (enums for short) are an odd variable type. They function as a list of possible values, and each value can be represented by its name or number. This allows them to be put in an order and compared with other values much like integers. As an example, if we had an enum called Altitude, we might write it like this:
enum EAltitude { ALT_Underground, ALT_Surface, ALT_Sky, ALT_Space, };
As we can see from the order these are in, ALT_Space
would be "greater than" ALT_Surface
if compared to it. Sometimes we might use enums where we don't care about the order. An example of this would be the EMoveDir
enum from Actor.uc
:
enum EMoveDir { MD_Stationary, MD_Forward, MD_Backward, MD_Left, MD_Right, MD_Up, MD_Down };
This enum describes the directions an actor can be moving, but in this case we only care that the variable can only have one value. The order of the elements doesn't matter in this case.
Enum values can also be used as bytes. If we looked at our example enum, this means that they would have the following values:
ALT_Underground = 0 ALT_Surface = 1 ALT_Sky = 2 ALT_Space = 3
Now the order makes sense, and we know why we can compare them to each other like integers. But then why not just use ints instead of enums to represent the values? For readability, mostly. Would you rather see that an actor's Altitude variable has been set to ALT_Sky
, or 2
?