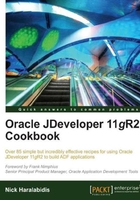
Overriding remove() to delete associated children entities
When deleting a parent entity, there are times you will want to delete all of the child entity rows in an entity assocation relation. In this recipe, we will see how to accomplish this task.
How to do it...
- Start by creating a new Fusion Web Application (ADF) workspace called HRComponents.
- Create a Database Connection for the
HR
schema in the Application Resource section of the Application Navigator. - Use the Business Components from Tables selection on the New Gallery dialog to create business components objects for the
DEPARTMENTS
andEMPLOYEES
tables. The components in the Application Navigator should look similar to the following: - Double-click on the EmpDeptFkAssoc association on the Application Navigator to open the association definition, then click on the Relationship tab.
- Click on the Edit accessors button (the pen icon) in the Accessors section to bring up the Association Properties dialog.
- Change the Accessor Name in the Destination Accessor section to DepartmentEmployees and click OK to continue.
- Double-click on the Department entity object in the Application Navigator to open its definition and go to the Java tab.
- Click on the Edit Java Options button (the pen icon on the top right of the tab) to bring up the Select Java Options dialog.
- On the Select Java Options dialog, select Generate Entity Object Class.
- Ensure that both the Accessors and Remove Method checkboxes are selected. Click OK to continue.
- Repeat steps 7-10 to create a Java implementation class for the
Employee
entity object. You do not have to click on the Remove Method checkbox in this case. - Open the
DepartmentImpl
Java implementation class for theDepartment
entity object in the JDeveloper Java editor and locate theremove()
method. - Add the following code before the call to
super.remove()
:// get the department employeess accessor RowIterator departmentEmployees = this.getDepartmentEmployees(); // iterate over all department employees while (departmentEmployees.hasNext()) { // get the department employee EmployeeImpl departmentEmployee =(EmployeeImpl)departmentEmployees.next(); // remove employee departmentEmployee.remove(); }
How it works...
During the creation of the Department
and Employee
entity objects, JDeveloper automatically creates the entity associations based on the foreign key constraints that exist among the DEPARTMENTS
and EMPLOYEES
database tables. The specific association that relates a department to its employees was automatically created and it was called EmpDeptFkAssoc
.
Jdeveloper exposes the association to both the source and destination entity objects via accessors. In step 6, we changed the accessor name to make it more meaningful. We called the the association accessor that returns the department employees DepartmentEmployees
. Using Java, this accessor is available in the DepartmentImpl
class by calling getDepartmentEmployees()
. This method returns an oracle.jbo.RowIterator
object that can be iterated over.
Now, let's take a closer look at the code added to the remove()
method. This method is called by the ADF framework each time we delete a record. In it, first we access the current department's employees by calling getDepartmentEmployees()
. Then we iterate over the department employees, by calling hasNext()
on the employees RowIterator
. Then for each employee, we get the Employee
entity object by calling next()
, and call remove()
on it to delete it. The call to super.remove()
finally deletes the Department
entity itself. The net result is to delete all employees associated with the specific department before deleting the department itself.
There's more...
A specific type of association called composition association can be enabled in those cases where an object composition behavior is observed, that is, where the child entity cannot exist on its own without the associated "parent" entity. In these cases, there are special provisions by the ADF framework and JDeveloper itself to fine-tune the delete behavior of child entities when the parent entity is removed. These options are available in the association editor Relationship tab, under the Behavior section.

Once you indicate a Composition Association for the association, two options are presented relating to cascading deletion:
- Optimize for Database Cascade Delete: This option prevents the framework from issuing a
DELETE
DML statement for each composed entity object destination row. You do this ifON DELETE CASCADE
is implemented in the database. - Implement Cascade Delete: This option implements the cascade delete in the middle layer, that is if the source composing entity object contains any composed children, its deletion is prevented.
This recipe shows how to remove children entity objects for which composition association is not enabled. This may be the case when a requirement exists to allow in some cases composed children entities to exist without associated composing parent entities. For example, when a new employee is not yet assigned to a particular department.