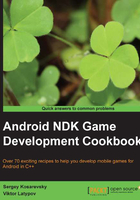
What this book covers
Chapter 1, Establishing a Build Environment, explains how to install and configure Android SDK and NDK on Microsoft Windows and Ubuntu/Debian Linux flavors, and how to build and run your first application on an Android-based device. You will learn how to use different compilers and toolchains that come with the Android NDK. Debugging and deploying the application using the adb tool is also covered.
Chapter 2, Porting Common Libraries, contains a set of recipes to port well-established C++ projects and APIs to Android NDK, such as FreeType fonts rendering library, FreeImage images loading library, libcurl and OpenSSL (including compilation of libssl and libcrypto), OpenAL API, libmodplug audio library, Box2D physics library, Open Dynamics Engine (ODE), libogg, and libvorbis. Some of them require changes to the source code, which will be explained. Most of these libraries are used later in subsequent chapters.
Chapter 3, Networking, shows you how to use the well-known libcurl library to download files using the HTTP protocol and how to form requests and parse responses from popular Picasa and Flickr online services directly using C++ code. Most applications nowadays use network data transfer in one way or another. HTTP protocol is the foundation of the APIs for all of the popular websites such as Facebook, Twitter, Picasa, Flickr, SoundCloud, and YouTube. The remaining part of the chapter is dedicated to the web server development. Having a mini web server in the application allows a developer to control the software remotely and monitor its runtime without using the OS-specific code. The beginning of the chapter also introduces a task queue for background download processing and simple smartpointers to allow efficient cross-thread data interchange. These threading primitives are used later on in Chapter 4, Organizing a Virtual Filesystem and Chapter 5, Cross-platform Audio Streaming.
Chapter 4, Organizing a Virtual Filesystem, is devoted entirely to the asynchronous file handling, resource proxies, and resource compression. Many programs store their data as a set of files. Loading these files without blocking the whole program is an important issue. Human interface guidelines for all modern operating systems prescript the application developer to avoid any delay, or "freezing", in the program's workflow (known as the Application Not Responding (ANR) error in Android). Android program packages are simply archive files with an .apk extension, compressed with a familiar ZIP algorithm. To allow reading the application's resource files directly from .apk, we have to decompress the .zip format using the zlib library. Another important topic covered is the virtual filesystem concept, which allows us to abstract the underlying OS files and folders structure, and share resources between Android and PC versions of our application.
Chapter 5, Cross-platform Audio Streaming, starts with organizing an audio stream using the OpenAL library. After this, we proceed to the RIFF WAVE file format reading, and OGG Vorbis streams decoding. Finally, we learn how to play some tracker music using libmodplug. Recent Android NDK includes an OpenSL ES API implementation. However, we are looking for a fully portable implementation between the desktop PC and other mobile platforms to allow seamless game debugging capabilities. To do this, we precompile an OpenAL implementation into a static library, and then organize a small multithreaded sound streaming library on top of libogg and libvorbis.
Chapter 6, Unifying OpenGL ES 3 and OpenGL 3, presents the basic rendering loop for the desktop OpenGL 3 and mobile OpenGL ES 3.0. Redeploying the application to a mobile device is a lengthy operation that prevents the developer from quick feature testing and debugging. In order to allow the development and debugging of game logic on the PC, we provide a technique to use desktop GLSL shaders in mobile OpenGL ES.
Chapter 7, Cross-platform UI and Input System, will teach you how to implement multi-touch event handling and gesture recognition in a portable way. A mobile is now almost synonymous with gesture-based touch input. No modern user-oriented application can exist without a graphical user interface (GUI). There are two basic issues to organize the interaction: input and text rendering. To ease the testing and debugging, we also show you how to simulate the multi-touch input on a Windows 7 PC equipped with multiple mouse devices. Since we are aiming at the development of interactive gaming applications, we have to implement user input in a familiar way. We will show you systematically how to create an on-screen gamepad UI. In a global multicultural environment, it is very desirable to have a multi-language text renderer for any application. We will show you how to use the FreeType library to render Latin, Cyrillic, and left-to-right texts. The organization of a multi-language UTF-8 localized interface will be presented as a dictionary-based approach.
Chapter 8, Writing a Match-3 Game, will put all the techniques we have introduced together, and write a simple Match-3 game, including rendering using OpenGL ES, input handling, resources packing, and PC-side debugging. The game is also runnable and debuggable on a Windows desktop PC and can be easily ported to other mobile platforms.
Chapter 9, Writing a Picture Puzzle Game, will provide a more complicated example, integrating all of the things mentioned above. All of the above elements regarding graphics and input will use native network libraries and APIs to download images from the Picasa online service.