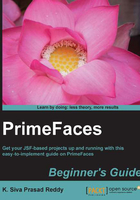
Time for action – creating a confirmation dialog
Let us see how to create a confirmation dialog with Yes and No options.
- Create a confirmation dialog using
<p:confirmDialog>
with two buttons for Yes and No options:<h:form id="form1" style="width: 300px; margin-left: 5px;"> <p:growl/> <p:panel header="User Form"> <h:panelGrid columns="2"> <h:outputText value="EmailId:" /> <p:inputText value="admin@gmail.com"/> <p:commandButton value="Delete" onclick="cnfDlg.show()" /> </h:panelGrid> </p:panel> <p:confirmDialog widgetVar="cnfDlg" header="Confirmation" message="Are you sure to delete?" > <p:commandButton value="Yes" actionListener="#{confirmDialogController.handleDelete}" oncomplete="cnfDlg.hide();" update="form1"/> <p:commandButton value="No" onclick="cnfDlg.hide();"/> </p:confirmDialog> </h:form>
- Implement the action handler method using the following code:
public void handleDelete(ActionEvent event) { FacesMessage msg = new FacesMessage(FacesMessage.SEVERITY_INFO, "Deleted Successfully",null); FacesContext.getCurrentInstance().addMessage(null, msg); }
What just happened?
We have created a ConfirmDialog
box using the <p:confirmDialog>
component with Yes and No buttons. As ConfirmDialog
is hidden by default, we have created a Delete button to display the confirmation dialog. When a Delete button is clicked, we will display the ConfirmationDialog
using a client-side JavaScript API call cnfDlg.show()
. When the Yes button is clicked, the action handler method handleDelete()
will be executed. Once the AJAX request is completed, the oncomplete
event callback gets invoked where we are closing the ConfirmationDialog
box using the cnfDlg.hide()
method. If the No button is clicked, then we are simply closing the Confirmation dialog box using the cnfDlg.hide()
method.
Using the global ConfirmDialog component
In our applications we might want to display confirmation dialogs with Yes or No options in many scenarios. Instead of creating a separate <p:confirmDialog>
component for each of the scenarios, we can use a single global confirm dialog box.
We can create a global ConfirmationDialog
as follows:
<p:confirmDialog global="true"> <p:commandButton value="Yes" type="button" styleClass="ui-confirmdialog-yes" icon="ui-icon-check"/> <p:commandButton value="No" type="button" styleClass="ui-confirmdialog-no" icon="ui-icon-close"/> </p:confirmDialog>
Note that in the global ConfirmDialog
component, the command component with styleClass="ui-confirmdialog-yes"
triggers a Yes action, and the command component with styleClass="ui-confirmdialog-no"
triggers a No action.
Once the global ConfirmDialog
component is defined, any trigger component, such as the Delete button in the preceding example, can use <p:confirm>
behavior by passing specific header text, dialog messages and icon values:
<p:commandButton value="Delete" actionListener="#{userController.deleteUser()}"> <p:confirm header="Confirmation" message="Are you sure to delete?" icon="ui-icon-alert"/> </p:commandButton>
Suppose you want to display a confirm dialog while deleting a Tag. We no longer need to create a <p:confirmDialog>
, instead we can just use <p:confirm>
to trigger the global ConfirmDialog
as follows:
<p:commandButton value="Delete" actionListener="#{adminController.deleteTag()}"> <p:confirm header="Confirmation" message="Are you sure to delete Tag?" icon="ui-icon-alert"/> </p:commandButton>
The global ConfirmDialog
component is very useful as we can define the global ConfirmDialog
component in the main layout page and can use <p:conrfirm>
to show ConfirmDialog
whenever required.