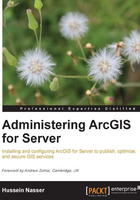
The classical web service
In a nutshell, a web service is a method that can be called by a client to perform a particular task and return some results. Such results can be in plain text, as links, or media that can be interpreted by browsers. What makes it different from any regular method is that this method is a cross platform, which means you can call it from practically anywhere and get the output in a convenient and native format. For example, you spend countless hours to develop an algorithm on C# that performs a certain task. Your boss is happy, and she/he asks you to deploy it on your Unix database server. However, there is a slight problem here. C# code uses the Microsoft .NET Framework that happens to run only on the Microsoft Windows operating system; therefore, it is a challenging task to port this algorithm to Unix. One solution will be to rewrite the algorithm to run on Unix, which will lead to two versions of the code scattered on multiple locations. However, this will introduce more maintenance work, which is not very efficient. Another solution is to deploy this algorithm as a web service, since web services use HTTP. You will be able to call this algorithm not only from Unix but also from practically any other platform.
It is a de facto that a web service should follow a known standard or protocol so clients can easily communicate with it and consume it. For instance, HTTP follows certain code for communicating between a client and server. You can choose to adopt this protocol and therefore all the clients who use HTTP can consume your service. There are cases where you want to create your own protocol. For instance, your web service contains sensitive data and supporting HTTP might compromise it. So your new protocol, let's call it X encrypts communications, so only clients who support protocol X can read this web service. I usually explain web service protocols by comparing it with the languages we speak. For example, I have chosen to write this book in the English language in favor of Arabic because English is a universal language and thus more readers would acquire this book. I can also write another edition of this book in Arabic, thus supporting two languages and increase my reader base.
Creating a classical web service
There are a number of protocols developed for web services. Before you go through these protocols, it is a good practice to know how classical web services work without a protocol. To tackle that, you will be creating your own raw web service from scratch. This way you will truly be able to grasp the idea behind web services' standards and then comfortably work with one without wondering about its mechanics. We will need a machine that runs IIS to do the following task. The machine WEB-SERVER01
that we created in the previous chapter will do fine.
Random numbers are used in so many applications. They are used during encryptions and for generating a passcode. In this exercise, we will create a sample web service that will return a random number.
In some cases, ASP might be turned off on the Web server, so we will make sure it is on. From the Server Manager window, select Roles and then Web Server (IIS). From the window on the right pane, scroll down and click on Add Roles Services. A new form will be displayed under the Application Development tree node; check the ASP box. Click on Next and then Install to install the required options for ASP.

Now we will start writing the web service. To do that, we will need to write some code. An artist needs a canvas to paint, and the canvas for programmers is the notepad. You may also use Notepad ++, Sublime Text, or your favorite editor. Click on Start and then type Notepad
. In the open Notepad, type the following code:
<% Response.Write ("Hello, World!") %>
The <%
and %>
tags indicate the opening and closing of the ASP code segment, Response.Write
is a function that will output a value to whoever is calling the service, and "Hello, World!"
is the string we want to output. This is just to test the service; we will change this later. Let's save this file at C:\inetpub\wwwroot
as genrand.asp
. Now open Chrome or any browser you have and type the following:
http://WEB-SERVER01/genrand.asp
This will output "Hello, World!" in the browser. Remember that you just called this service from the browser, so you will get the output there. If you called it from within a C++ application, Python script, or a terminal, you will get the result there. Let's refine the code, open our file again in Notepad, erase everything, and write the following code instead:
<% Dim r r = Rnd() r = r * 100 r = Round(r) Response.Write(r) %>
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www. packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Save the file and run it again. You will get a random number. I got 71, and yours might be different. Try running the service again; you will get the exact same number. Why is that? The problem with generating random numbers with computers is that computers are predicable. They are machines that we program. Computers cannot just think of a number. It should come from somewhere, either from a time stamp or from an external value such as the weather temperature. If I asked you to think of a number, you will give me a different number every time. This is why hackers with experience can predict what the computer will do next if they could understand how the random generator algorithm is coded. The reason you are getting the same number is that every time you call this service, you initiate a new process that is not associated with the previous call. It happens that the first random number in my case is always 71. This is why you keep getting the same number every time you refresh the page, not so random. To create a real random number, we should seed it with something slightly unpredictable, and nothing is more unpredictable than nature. You could read different information on the weather such as wind velocity, temperature, and so on, and toss this data to create a random number. However, for the sake of this exercise, we will seed it with less predictable data, the time.
Let's open our file again and add the highlighted code shown as follows:
<%
Dim r
Randomize (Timer)
r = Rnd()
r = r * 100
r = Round(r)
Response.Write(r)
%>
Open your service again. Now you will start getting some different numbers. You can start adding more complexity to this service to generate even more random or even more unique numbers.
You might say this seems like a normal web page and that is true. However, instead of returning HTML content, this page returns a single number. So we computer scientists decided to give it another name, a web service, to differentiate it from the classical web page. For instance, consider the Extensible Markup Language (XML) and HTML languages. Both of them are markup languages at their cores. The difference is that HTML is a special case of XML with special tags that follow a standard. The Geographic Markup Language (GML) falls in the same category, a markup language with special geography tags. You can say that a web page is a special case of a web service that returns, well, a page.
Now that you know how a web service works, consider Twitter API, Facebook API, or ArcGIS API; all of these are web services exposed in the same way you just did. The only difference is that they follow a set of standards that make them universal and easier to consume.