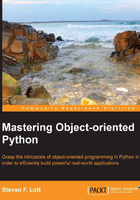
Extending a collection class
An alternative to wrapping is to extend a built-in class. By doing this, we have the advantage of not having to reimplement the pop()
method; we can simply inherit it.
The pop()
method has the advantage that it creates a class without writing too much code. In this example, extending the list
class has the disadvantage that this provides many more functions than we truly need.
The following is a definition of Deck
that extends the built-in list
:
class Deck2( list ): def __init__( self ): super().__init__( card6(r+1,s) for r in range(13) for s in (Club, Diamond, Heart, Spade) ) random.shuffle( self )
In some cases, our methods will have to explicitly use the superclass methods in order to have proper class behavior. We'll see other examples of this in the following sections.
We leverage the superclass's __init__()
method to populate our list
object with an initial single deck of cards. Then we shuffle the cards. The pop()
method is simply inherited from list
and works perfectly. Other methods inherited from the list
also work.