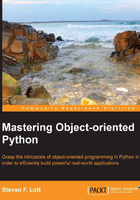
上QQ阅读APP看书,第一时间看更新
Simplicity and consistency using elif sequences
Our factory function, card()
, is a mixture of two very common factory design patterns:
- An
if-elif
sequence - A mapping
For the sake of simplicity, it's better to focus on just one of these techniques rather than on both.
We can always replace a mapping with elif
conditions. (Yes, always. The reverse is not true though; transforming elif
conditions to a mapping can be challenging.)
The following is a Card
factory without the mapping:
def card3( rank, suit ): if rank == 1: return AceCard( 'A', suit ) elif 2 <= rank < 11: return NumberCard( str(rank), suit ) elif rank == 11: return FaceCard( 'J', suit ) elif rank == 12: return FaceCard( 'Q', suit ) elif rank == 13: return FaceCard( 'K', suit ) else: raise Exception( "Rank out of range" )
We rewrote the card()
factory function. The mapping was transformed into additional elif
clauses. This function has the advantage that it is more consistent than the previous version.