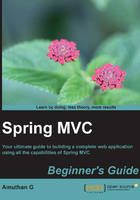
Time for action – examining request mapping
Let's observe what will happen when you change the value
attribute of the @RequestMapping
annotation by executing the following steps:
- Open your STS and run the webstore project; just right-click on your project and choose Run As | Run on Server. You will be able to view the same welcome message on the browser.
- Now, go to the address bar of the browser and enter the URL,
http://localhost:8080/webstore/welcome
. - You will see the HTTP Status 404 error page on the browser, and you will also see the following warning in the console:
WARNING: No mapping found for HTTP request with URI [/webstore/welcome] in DispatcherServlet with name ' DefaultServlet'
An error log displaying the "No mapping found" warning message
- Now, open the
HomeController
class, change the@RequestMapping
annotation'svalue
attribute to/welcome
, and save it. Basically, your new request mapping annotation will look like@RequestMapping("/welcome")
. - Again, run the application and enter the same URL that you entered in step 2; now you will be able to see the same welcome message on the browser, without any request mapping error.
- Finally, open the
HomeController
class and revert the changes that were made to the@RequestMapping
annotation's value; just make it@RequestMapping("/")
again and save it.
What just happened?
After starting our application, when we enter the URL http://localhost:8080/webstore/welcome
on the browser, the dispatcher servlet (org.springframework.web.servlet.DispatcherServlet
) immediately tries to find a matching controller method for the request path, /welcome
.
Tip
In a Spring MVC application, the URL can logically be divided into five parts (see the following figure); the @RequestMapping
annotation only matches against the URL request path. It omits the scheme, hostname, application name, and so on.
The @RequestMapping
annotation has one more attribute called method
to further narrow down the mapping based on the HTTP request method types (GET
, POST
, HEAD
, OPTIONS
, PUT
, DELETE
, and TRACE
). If we do not specify the method
attribute in the @RequestMapping
annotation, the default method will be GET
. We will learn more about the method
attribute of the @RequestMapping
annotation in Chapter 4, Working with Spring Tag Libraries, under the section on form processing.

The logical parts of a typical Spring MVC application URL
Since we don't have a corresponding request mapping for the given URL path, /welcome
, we get the HTTP Status 404 error on the browser and the following error log on the console:
WARNING: No mapping found for HTTP request with URI [/webstore/welcome] in DispatcherServlet with name 'DefaultServlet'
From the error log, we can clearly understand that there is no request mapping for the URL path, /webstore/welcome
. So, we try to map this URL path to the existing controller method; that's why, in step 4, we put only the request path value, /welcome
, in the @RequestMapping
annotation as the value
attribute. Now everything works perfectly fine.
Finally, we reverted our @RequestMapping
annotation's value to /
again in step 6. Why did we do this? Because we want it to show the welcome page under the web request URL http://localhost:8080/webstore/
again. Observe carefully that here the last single character /
is the request path. We will see more about request mapping in upcoming chapters.
Pop quiz – request mapping
Q1. If we have a Spring MVC application for library management called BookPedia and want to map a web request URL, http://localhost:8080/BookPedia/category/fiction
, to a controller method, how will we form the @RequestMapping
annotation?
@RequestMapping("/fiction")
.@RequestMapping("/category/fiction")
.@RequestMapping("/BookPedia/category/fiction")
.