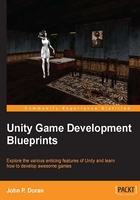
Customizing the GUI
While it is great that Unity provides us with all of this functionality to save us time in creating elements, the actual aesthetics leaves a little to be desired. Thankfully, UnityGUI allows us to customize the appearance of our controls by making use of GUIStyle
, which is an optional third parameter to our control functions. If we do not specify a GUIStyle
parameter, Unity's default will be used, which is what we experienced last time. This can work fine while testing something out. However, since we're trying to create a polished and complete project, we're going to create one of our own by performing the following steps:
- Open up the
MainMenuGUI
script file and modify the function to accommodate the changes in bold:using UnityEngine; using System.Collections; public class MainMenuGUI : MonoBehaviour { public int buttonWidth = 100; public int buttonHeight = 30; public GUIStyle titleStyle; public GUIStyle buttonStyle; void OnGUI() { //Get the center of our screen float buttonX = ( Screen.width - buttonWidth ) / 2.0f; float buttonY = ( Screen.height - buttonHeight ) / 2.0f; //Show button on the screen and check if clicked if ( GUI.Button( new Rect( buttonX, buttonY, buttonWidth, buttonHeight ), "Start Game", buttonStyle ) ) { // If button clicked, load the game level Application.LoadLevel("Chapter_1"); } // Add game's title to the screen, above our button GUI.Label( new Rect(buttonX + 2.5f , buttonY - 50, 110.0f, 20.0f), "Twinstick Shooter", titleStyle ); } }
- Once finished, save the file into your
Scripts
folder and then move back into Unity. You should see the two variables showing up in the Inspector section: - For the Title Style option, navigate to Normal | Text Color and put in
white
. Change the Font option to the OSP-DIN font we added in the previous chapter, the Font Size option to42
, and the Alignment option to Middle Center: - Now, for the
buttonStyle
variable (Button Style in the inspector), we're first going to need some additional images for our buttons. So, go into yourChapter 2/Assets
location, which you should have obtained from Packt Publishing's website, and drag-and-drop the new images into ourSprites
folder. - Once that's finished, set the Normal, Hover, and Active Background properties to the blue, yellow, and green buttons, respectively. Next, set the Border property to
10
in each of the four directions. Setting the border will make sure that the background images do not exceed those many pixels in each of those directions. This is great for objects that can be different sizes, such as buttons and text fields. Finally, set the Font option to OSP-DIN and the Alignment option to Middle Center: - With all of that done, save your scene and run the game as shown in the following screenshot:
We now have something that already looks a lot better than what we had before, and you learned how to work with different styles!