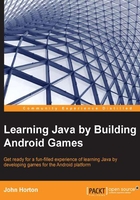
Our first look at Java
So what about all that code that Android Studio generated when we created our new project earlier? This is the code that will bring our game menu to life. Let's take a closer look. The very first line of code in the editor window is this:
package com.packtpub.mathgamechapter2;
This line of code defines the package that we named when we first created the project. As the book progresses, we will write more complex code that spans more than one file. All the code files we create will need the package they belong to, clearly defined like the previous line of code, at the top. The code doesn't actually do anything in our game. Notice also that the line ends with a semicolon (;
). This is a part of the Java syntax and it denotes the end of a line of code. Remove a semicolon and you will get an error because Android Studio tries to make sense of two lines together. Try it if you like.
Tip
Remember that if you are going to be copying and pasting the code from the download bundle, this is the one line of code that might vary depending on how you set up your project. If the package name in the code file is different from the package name you created, always use the package name from when you created the project.
To see the next four lines of code, you might need to click on the small + icon to reveal them. Android Studio tries to be helpful by simplifying our view of the code. Notice that there are several little - icons as well down the side of the editor window. You can expand and collapse them to suit yourself without affecting the functionality of the program. This is shown in the following screenshot:

Once you have expanded the code, you will see these four lines:
import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem;
Notice that all the preceding lines start with the word import
. This is an instruction to include other packages in our game, not just our own. This is very significant because it makes available to us all of the hard work of other programmers, the Android development team in this case. It is precisely these imports that give us the ability to use the methods we discussed earlier, and allow us to interact with the Android life cycle phases. Notice again that all the lines end with a semicolon (;
).
The next line introduces a fundamental building block of Java known as a class. Classes are something that we will continually expand our knowledge and understanding of throughout the book. For now, take a look at this line of code, then we will discuss it in detail:
public class MainActivity extends ActionBarActivity {
Word by word, here is what is going on. The preceding line is saying: make me a new public class
called MainActivity
and base it upon (extends
) ActionBarActivity
.
You might remember that MainActivity
is the name we chose while creating this project. ActionBarActivity
is the code (known as a class) written by the Android development team that enables us to put our Java into Android.
If you have a keen eye, you might notice there is no semicolon at the end of this line. There is, however, an opening curly brace ({
). This is because MainActivity
encompasses the rest of the code. In effect, everything is part of our MainActivity
class, which is built based on the ActionBarActivity
class/code. If you scroll down to the bottom of the editor window, you will see a closing curly brace (}
). This denotes the end of our class called MainActivity
.
- We do not need to know how a class works yet
- We will use classes to access some methods contained within its code and without doing any more, we are already, by default, taking advantage of the Android life cycle methods we discussed earlier
- We can now pick and choose if, when, and which methods defined in these classes we wish to override or leave as default
So, it is the ActionBarActivity
class that contains the methods that enable us to interact with the Android life cycle. Actually, there are a number of different classes that enable us to do this and in a moment, we will change from using ActionBarActivity
to a more appropriate class that also does all the things just mentioned.
Tip
It is not important at this point to properly understand Java classes; just understand that you can import a package and a package can contain one or more classes that you can then use the functionality of or base your own Java programs on.
We will bump into classes regularly in the next few chapters. Think of them as programming black boxes that do stuff. In Chapter 6, OOP – Using Other People's Hard Work, we will open the black box and really get to grips with them and we will even start making our own classes.
Moving on with the code, let's look at what the code that is contained within our class actually does.
Here is the code chunk directly after the crucial line we have just been discussing:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); }
Hopefully, some of this code will start to make sense now and tie in with what we have already discussed. Although the precise syntax will still feel a little alien, we can continue learning Java as long as we are aware of what is happening.
The first thing we notice in the preceding code is the word @override
. Remember when we said that all the methods that interact with the Android life cycle were implemented by default and we can pick and choose if and when to override them? This is what we are doing here with the onCreate
method.
The @override
word says that the method that follows next is being overridden. The protected void onCreate(Bundle savedInstanceState) {
line contains the method we are overriding. You might be able to guess that the action starts with the opening {
at the end of the line in question and ends with the closing }
three lines later.
The somewhat odd-looking protected void
before the method name onCreate
and (Bundle savedInstanceState)
after the method name are unimportant at this time because they are handled for us. It is to do with the data that travels between various parts of our program. We just need to know that what happens here will take place in the creating phase of the Android lifecycle. The rest will become clear in Chapter 4, Discovering Loops and Methods. Let's move on to the line:
super.onCreate(savedInstanceState);
Here, the super
keyword is referencing the code in the original onCreate
method, which is still there even though we can't see it. The code is saying: even though I am overriding you, I want you to set things up, just like you normally do first. Then, after onCreate
has done loads of work that we don't see and don't need to see, the method continues and we actually get to do something ourselves with this line of code:
setContentView(R.layout.activity_main);
Here we are telling Android to set the main content view (our users screen), which is the cool game menu we created earlier. To be specific, we are stating it is an R
or resource in the layout
folder and the file is called activity_main
.
Cleaning up our code
The next two blocks of code were created by Android Studio on the assumption that we would want to override another two methods. We don't, because they are methods more often used in non-gaming apps:
- Delete the entire content shown in the following code. Be careful not to delete the closing curly brace of our
MainActivity
class:@Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); }
- Now we can delete a couple of the
@import
statements. The reason for this is that we just deleted the overridden methods of classes (imported earlier) we no longer need. Notice that the following lines in the editor window are grey. Note that the program would still work if you leave them in. Delete them both now to make your code as clear as possible:import android.view.Menu; import android.view.MenuItem;
- Some final amendments before our code is done: at this point, you might be thinking that we have deleted and changed so much of our code that we might as well have started from an empty page and typed it in. This is almost true. But the process of having Android Studio create a new project for us and then making these amendments is more thorough and also avoids quite a few steps. Here are the last code changes. Change the
import android.support.v7.app.ActionBarActivity;
line toimport android.support.app.Activity;
. - Now you will get several red lines underlining our code and indicating errors. This is because we are attempting to use a class we have not yet imported. Simply amend the
public class MainActivity extends ActionBarActivity {
line topublic class MainActivity extends Activity {
.
What we did with those last two changes was using a slightly more appropriate version of the Activity
class. To do this, we also had to change what we imported.
When you're done, your editor window should look exactly like this:
package com.packtpub.mathgamechapter2.mathgamechapter2; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; public class MainActivity extends ActionBarActivity { @Override protected void onCreate(Bundle savedInstancePhase) { super.onCreate(savedInstancePhase); setContentView(R.layout.activity_main); } }
Tip
Downloading the example code
You can download the example code fies from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the fies e-mailed directly to you.
Now that we know what's going on and our code is clean and lean, we can actually take a look at the beginnings of our game in action!
Tip
If any of what we have just discussed seemed complicated, there is no need for concern. Android forces us to work within the Activity lifecycle, so the previous steps were unavoidable. Even if you didn't follow all the explanations about classes and methods and so on, you are still perfectly placed to learn Java from here. All the classes and methods will seem much more straightforward as the book progresses.