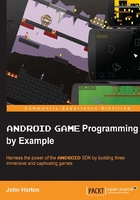
The thrill of flight – scrolling the background
Implementing our space dust is going to be really quick and easy. All we will do is create a SpaceDust
class with very similar properties to our other game objects. Spawn them into the game at a random location, move them toward the player at a random speed, and respawn them on the far right of the screen, again with a random speed and y coordinate.
Then in our TDView
class, we can declare a whole array of these objects, update, and draw them each frame.
Create a new class and call it SpaceDust
. Now enter this code:
public class SpaceDust { private int x, y; private int speed; // Detect dust leaving the screen private int maxX; private int maxY; private int minX; private int minY; // Constructor public SpaceDust(int screenX, int screenY){ maxX = screenX; maxY = screenY; minX = 0; minY = 0; // Set a speed between 0 and 9 Random generator = new Random(); speed = generator.nextInt(10); // Set the starting coordinates x = generator.nextInt(maxX); y = generator.nextInt(maxY); } public void update(int playerSpeed){ // Speed up when the player does x -= playerSpeed; x -= speed; //respawn space dust if(x < 0){ x = maxX; Random generator = new Random(); y = generator.nextInt(maxY); speed = generator.nextInt(15); } } // Getters and Setters public int getX() { return x; } public int getY() { return y; } }
Here is what is happening in the SpaceDust
class. At the top of the previous block of code, we declare our usual speed and maximum and minimum variables. They will allow us to detect when the SpaceDust
object leaves the left of the screen and needs respawning on the right, and provide sensible bounds for the height at which we respawn the object.
Then inside the SpaceDust
constructor, we initialize the speed
, x
, and y
variables with random values, but within the bounds set by the maximum and minimum variables we just initialized.
Then we implement the SpaceDust
class's update
method, which moves the object to the left based on the speed of the object and the player, then checks if the object has flown of the left-hand edge of the screen and respawns it with random but appropriate values if it has.
At the bottom, we provide two getter methods so that our draw
method knows where to draw each speck of dust.
Now, we can create an ArrayList
object to hold all our SpaceDust
objects. Declare it just under the declaration of the other game objects near the top of the TDView
class:
// Make some random space dust public ArrayList<SpaceDust> dustList = new ArrayList<SpaceDust>();
In the TDView
constructor, we can initialize a whole bunch of the SpaceDust
objects using a for
loop and then stash them into the ArrayList
object:
int numSpecs = 40; for (int i = 0; i < numSpecs; i++) { // Where will the dust spawn? SpaceDust spec = new SpaceDust(x, y); dustList.add(spec); }
We create forty specks of dust in total. Each time through the loop, we create a new speck of dust and the SpaceDust
constructor assigns it a random location and a random speed. We then put the SpaceDust
object into our ArrayList
object with dustList.add(spec);
Next, we jump to our TDView
class's update
method and use an enhanced for
loop to call update()
on each of our SpaceDust
objects:
for (SpaceDust sd : dustList) { sd.update(player.getSpeed()); }
Remember that we passed in the player speed so that the dust increases and decreases its speed relative to the player's speed.
Now to draw all our space dust, we loop through our ArrayList
object and draw a speck at a time. Of course, we add the code to our TDView
class's draw
method, but we must make sure to draw the space dust first so it appears behind the other game objects. In addition, we have an extra line to switch pixel color to white before using the drawPoint
method of our Canvas
object to plot a single pixel for each SpaceDust
object.
In the draw
method of the TDView
class, add this code to draw our dust:
// White specs of dust paint.setColor(Color.argb(255, 255, 255, 255)); //Draw the dust from our arrayList for (SpaceDust sd : dustList) { canvas.drawPoint(sd.getX(), sd.getY(), paint); // Draw the player // ... }
The only new thing here is the canvas.drawpoint...
line of code. Apart from drawing bitmaps to the screen, the Canvas
class allows us to draw primitives, like points and lines, as well as things like text and shapes. We will use these features when drawing a HUD for our game in Chapter 4, Tappy Defender – Going Home.
Why not run the app and check out how much neat stuff we have implemented? In this screenshot, I have temporarily increased the number of the SpaceDust
objects to 200
, just for fun. You can also see that we have enemies drawn, attacking at a random y coordinate with random speed:
