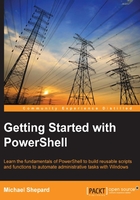
Objects all the way down
One major difference between PowerShell and other command environments is that, in PowerShell, everything is an object. One result of this is that the output from the PowerShell cmdlets is always in the form of objects. Before we look at how this affects PowerShell, let's take some time to understand what we mean when we talk about objects.
Digging into objects
If everything is an object, it's probably worth taking a few minutes to talk about what this means. We don't have to be experts in object-oriented programming to work in PowerShell, but a knowledge of a few things is necessary.
In a nutshell, object-oriented programming involves encapsulating the related values and functionality in objects. For instance, instead of having variables for speed, height, and direction and a function called PLOTPROJECTILE
, in object-oriented programming you might have a Projectile object that has the properties called Speed
, Height
, and Direction
as well as a method called Plot
. This Projectile object could be treated as a single unit and passed as a parameter to other functions. Keeping the values and the related code together has a certain sense to it, and there are many other benefits to object-oriented programming.
Types, classes, and objects
The main concepts in object-oriented programming are types, classes, and objects. These concepts are all related and often confusing, but not difficult once you have them straight:
- A type is an abstract representation of structured data combining values (called properties), code (called methods), and behavior (called events). These attributes are collectively known as members.
- A class is a specific implementation of one or more types. As such, it will contain all the members that are specified in each of these types and possibly other members as well. In some languages, a class is also considered a type. This is the case in C#.
- An object is a specific instance of a class. Most of the time in PowerShell, we will be working with objects. As mentioned earlier, all output in PowerShell is in the form of objects.
PowerShell is build upon the .NET framework, so we will be referring to the .NET framework throughout this book. In the .NET framework, there is a type called System.IO.FileSystemInfo
, which describes the items located in a filesystem (of course). Two classes that implement this type are System.IO.FileInfo
and System.IO.DirectoryInfo
. A reference to a specific file would be an object that was an instance of the System.IO.FileInfo
class. The object would also be considered to be of the System.IO.FileSystemInfo
and System.IO.FileInfo
types.
Here are some other examples that should help make things clear:
- All the objects in the .NET ecosystem are of the
System.Object
type - Many collections in .NET are of the
IEnumerable
type - The ArrayList is of the
IList
andICollection
types among others