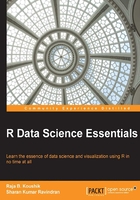
Control structures in R
We have covered the different operations that are available in R. Now, let's look at the control structures used in R. Control structures are the key elements of any programming language.
The control structures commonly used in R are as follows:
if
,else
: This is used to test a condition and execute based on the conditionfor
: This is used to execute a loop for a fixed number of iterationswhile
: This is used to execute a loop while a condition is truerepeat
: This is used to execute a loop indefinitely until seeking a breakbreak
: This is used to break the execution of a loopnext
: This is used to skip an iteration of a loopreturn
: This is used to exit a function
Control structures – if and else
The if
and else
control structures are used to execute based on a condition, where it performs the function when the condition is satisfied and performs an alternate function when the condition fails. (The else clause is not mandatory.) We can implement nested conditions as well in R.
if(<condition>) { ## do something } else { ## do something else }
Control structures – for
The for
loop is used to execute repetitive code statements for a definite number of iterations. The for
loops are commonly used to iterate over the element of an object (list, vector, and so on).
for(i in 1:10) { print(i) }
Control structures – while
The while
loops are used to evaluate a condition repetitively. If the condition is true, then the expression in the loop body is executed until the condition becomes false.
count<-0 while(count<10) { print(count) count<-count+1 }
Control structures – repeat and break
The repeat
statement executes an expression in the loop repeatedly until it encounters a break.
The break
statement can be used to terminate any loop. It is the only way to terminate a repeat loop.
> sum <- 1 > repeat { sum <- sum + 2; print(sum); if (sum > 11) break; } 3 5 7 9 11 13
Control structures – next and return
The next
control structure is used to skip a particular iteration in a loop based on a condition.
The return
control structure signals that a function should exit a function and return a given value.
for(i in 1:5) { if(i<=3) { next } print(i) } [1] 4 [1] 5