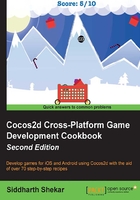
Adding a MainMenu Scene
To create the main menu scene, first create a new project and follow the steps in Chapter 1, Sprites and Animations, to load the MainScene
file upon loading the game. Also, don't forget to make changes to the CCBReader.m
file and change the folder search parameter.
Getting ready
For this step, no additional steps are required. Later in the chapter, we will discuss how to make more complex scenes with the custom init function.
For now, we will use MainScene
as the MainMenu
scene and start populating it with text, buttons, and functions to transition between the scenes.
How to do it…
As of now, we have a MainMenu.h
file that looks similar to the following:
@interface MainScene : CCNode +(CCScene*)scene; @end
We also have a MainScene.m
file, as follows:
#import "MainScene.h" @implementation MainScene +(CCScene*)scene{ return[[self alloc]init]; } -(id)init{ if(self = [super init]){ winSize = [[CCDirector sharedDirector]viewSize]; } return self; } @end
There is nothing new here. We can add the background image as we did in the first chapter in the init
function to get the scene to look better. We will add the following code to the init
function:
-(id)init{ if(self = [super init]){ winSize = [[CCDirector sharedDirector]viewSize]; CCSprite* backgroundImage = [CCSprite spriteWithImageNamed:@"Bg.png"]; backgroundImage.position = CGPointMake(winSize.width/2, winSize.height/2); [self addChild:backgroundImage]; } return self; }
How it works…
Build and run it to make sure that there are no errors because we will use this file as the main menu scene.
After running the scene, you will see the background image that you had learned to add in Chapter 1, Sprites and Animations.
Scenes are building blocks for creating interfaces for any games. A scene may contain any number of sprites, text labels, menus, and so on in any combination, and it is up to the developer's needs to populate a scene with these elements.
This is just to show how scenes are created and how the basic game scene looks. Later in the chapter, we will discuss how to customize our scenes.

Now, we will add text labels, buttons, and menus, and start populating our main menu scene next.