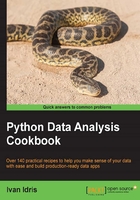
上QQ阅读APP看书,第一时间看更新
Creating heatmaps
Heat maps visualize data in a matrix using a set of colors. Originally, heat maps were used to represent prices of financial assets, such as stocks. Bokeh is a Python package that can display heatmaps in an IPython notebook or produce a standalone HTML file.
Getting ready
I have Bokeh 0.9.1 via Anaconda. The Bokeh installation instructions are available at http://bokeh.pydata.org/en/latest/docs/installation.html (retrieved July 2015).
How to do it...
- The imports are as follows:
from collections import OrderedDict from dautil import data from dautil import ts from dautil import plotting import numpy as np import bokeh.plotting as bkh_plt from bokeh.models import HoverTool
- The following function loads temperature data and groups it by year and month:
def load(): df = data.Weather.load()['TEMP'] return ts.groupby_year_month(df)
- Define a function that rearranges data in a special Bokeh structure:
def create_source(): colors = plotting.sample_hex_cmap() month = [] year = [] color = [] avg = [] for year_month, group in load(): month.append(ts.short_month(year_month[1])) year.append(str(year_month[0])) monthly_avg = np.nanmean(group.values) avg.append(monthly_avg) color.append(colors[min(int(abs(monthly_avg)) - 2, 8)]) source = bkh_plt.ColumnDataSource( data=dict(month=month, year=year, color=color, avg=avg) ) return year, source
- Define a function that returns labels for the horizontal axis:
def all_years(): years = set(year) start_year = min(years) end_year = max(years) return [str(y) for y in range(int(start_year), int(end_year), 5)]
- Define a plotting function for the heat map that also sets up hover tooltips:
def plot(year, source): fig = bkh_plt.figure(title="De Bilt, NL Temperature (1901 - 2014)", x_range=all_years(), y_range=list(reversed(ts.short_months())), toolbar_location="left", tools="resize,hover,save,pan,box_zoom,wheel_zoom") fig.rect("year", "month", 1, 1, source=source, color="color", line_color=None) fig.xaxis.major_label_orientation = np.pi/3 hover = fig.select(dict(type=HoverTool)) hover.tooltips = OrderedDict([ ('date', '@month @year'), ('avg', '@avg'), ]) bkh_plt.output_notebook() bkh_plt.show(fig)
- Call the functions you defined:
year, source = create_source() plot(year, source)
Refer to the following plot for the end result:

The source code is available in the heat_map.ipynb
file in this book's code bundle.
See also
- The Bokeh documentation about embedding Bokeh plots at http://bokeh.pydata.org/en/latest/docs/user_guide/embed.html (retrieved July 2015)