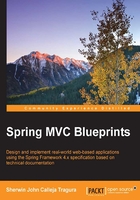
Technical requirement
This project will use the same installers and tools listed in Chapter 1, Creating a Personal Web Portal (PWP), but there are some additional components and libraries that are required to be part of the STS project.
The Maven dependencies that need to be added are the following:
- commons-fileupload: This dependency consists of classes and interfaces needed to create an easy, robust, and high-performance, file upload capability in servlet-based web applications; this library parses HTTP submitted using the POST method, and with a content type of "multipart/form-data", and exposes the polished request to the caller.
- commons-io: This dependency consists of classes and interfaces that support file reading, writing and other management transactions.
- commons-net: This dependency consists of a set of classes and interfaces that provide a fundamental protocol access to establish handshake transactions like FTP/FTPS access.
The library's commons-fileupload and commons-io are needed for the file uploading and downloading, while the commons-net are needed for dropping and retrieving files into an FTP server.
Since AJAX transactions will be part of the prototype, the following JavaScript libraries need to be included in the project:
jquery-2.1.4.min.js
: The compressed version of the JQuery library; JQuery is a JavaScript library that can manipulate HTML, DOM, and CSS, handle HTML events, can implement AJAX transactions and provides other utilities like animations and effects.jquery.form.min.js
: This is a JavaScript library that processes form elements in an HTML page.
The preceding JavaScript libraries are separately used to implement uploading of files using the AJAX mechanism.
The pom.xml
The pom.xml
file still consists of the core Spring MVC components of Chapter 1, Creating a Personal Web Portal (PWP), with the addition of the following dependencies:
<dependency> <groupId>commons-net</groupId> <artifactId>commons-net</artifactId> <version>3.3</version> </dependency> <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>1.3.1</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.2</version> </dependency>
The process flow
The following is an overview of the design:

The EDMS starts when the user clicks any of the three following options for uploading:
- Upload to Repository: This creates a copy of the uploaded file and stores it in the hard disk with or without encryption.
- Upload to FTP Server: This retrieves the file from a fixed local storage, and then creates a copy of it in the FTP server location. In order to copy the file, the user needs to input a valid username and password into the FTP server machine.
- Intranet Upload: This allows the user to upload the file via a JavaScript client code, and then store the file locally, just like a typical upload to repository strategy.
These three transactions can upload single or multiple documents as desired by the user.
After the form submission, the request will be parsed by the commons-upload libraries, then map the multipart/form-data object to the MultipartFile implementation. The handler method assigned to the request can now extract the file uploaded through the MultipartFile, and eventually store the object into the file system, or to the FTP server, using commons-io and old IO API classes.
Once uploaded, EDMS has the capability to read all uploaded documents in order for the user to download them again. Either the documents are stored in the file system, or the FTP server, controllers can retrieve them and map them on a view that can provide the download links per file.
The discussion will revolve around the details of how to set up a document library module, like EDMS, using the Spring MVC 4.x specification.