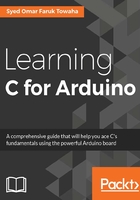
Collecting and showing data through serial port
Before going any further, let's discuss how we can print something using Arduino IDE. We already printed Hello Arduino in the previous chapter. If you don't know how to print something using serial monitor, please go to the previous chapter and then come back here.
Let's recap the print program we used.
We took two functions, setup()
and loop()
. Inside the setup function, we declared our baud rate as follows:
Serial.begin(9600); //default baud for serial communication
Right after the baud was defined, we added a special function to print our text on the serial monitor. The function was Serial.print()
. Inside the print()
function, we wrote (in C we call it passed ) what we wanted to be seen on the serial monitor.
So, the full code for printing Hello Arduino is as follows:
void setup(){ Serial.begin(9600); Serial.print("Hello Arduino"); } void loop() { }
You may know the difference between Serial.print()
and Serial.println()
. Serial.print()
prints a text on Serial Monitor
without a new line after the text, but Serial.println()
adds a new line after the text is printed. The following screenshot will help you understand the difference between the two functions:

In the preceding screenshot, you can see I printed three Serial.print()
functions, one after another, but on the serial monitor, they printed on a single line. I also printed three Serial.println()
functions, and they were printed as we wanted. We can also use '\n' at the end of our text on the Serial.print()
function, which will do the same thing as the Serial.println()
function. From the previous chapter, we know that \n
is called an escape sequence.
Let's define a few integer variables and print them on serial monitor. Look at the following code, where we assigned two integer variables, myNumber01
and myNumber02
, and printed them using the Serial.println()
function:
int myNumber01 = 1200; void setup() { int myNumber02 = 1300; Serial.begin(9600); Serial.println(myNumber01); Serial.println(myNumber02); } void loop() { }
Have you noticed I declared the variables in two places? One is declared before the setup()
function, and another inside the setup()
function. Amazingly, both are working. Ok, let's make it clear. The myNumber01
variable is called a global variable because it is not inside a function. If any variable is inside a function, it is called a local variable and it is available only in that function. So, myNumber02
is a local variable, as it is inside the setup()
function. Let's look at the output of the preceding code:

If we do not want to print a text directly from the print
function, we do not use quotes inside the print
function. So, the following code will give you different outputs:
int myNumber01 = 1200; void setup() { int myNumber02 = 1300; Serial.begin(9600); Serial.println(myNumber01); Serial.println("myNumber02"); //Just added quotations } void loop() { }
The output of the code will look like the following screenshot:

Let's print a float and a double number. The code is as follows:
double pie = 3.14159; float CGPA = 3.9; void setup() { Serial.begin(9600); Serial.println(pie); Serial.println(CGPA); } void loop() { }
The output will be similar to the following screenshot:

If you carefully look at the output, you will see the output of a double number is not exactly what we wrote. On Arduino Uno, float and double numbers are basically the same while printed via the print()
function. The print()
function prints only two decimal digits by default. Both float and double can hold the input of two digits after the decimal. Since both have a limit to hold a number, let's assign some long number to a double variable and see what happens. Perhaps your Arduino gets blasted. Just kidding. Let's look at what happens on our output. The code is as follows:
double longNumber= 847982635862956295.982378295327; double negativeLongNumber = -947973249864967657.9304709372409832; void setup() { Serial.begin(9600); Serial.println(longNumber); Serial.println(negativeLongNumber); } void loop() { }
The output will be similar to the following screenshot:

By ovf
,
we mean overflow. This means the number we assigned to our variables is larger than the limit of the data type.
The same thing happens with the integer data type. Can you check it at home? Don't worry, your Arduino won't burn.
Let's take the input of some data types.
If we want to take input from a keyboard, we need to check if there is available data incoming from the serial monitor. To check this, we need to use a condition. For now, let's remember to include the following line after your baud rate (it can be inside the loop()
function too, if you want to repeatedly check the serial and want to send data):
while (Serial.available() == 0);
Now, let's declare a global variable. In my case, it is as follows:
int letmego;
After the code, while (Serial.available() == 0);
, write the following code:
letmego = Serial.parseInt();
The Serial.parseInt()
function will convert the received byte from the serial monitor into an integer and store it to our variable. Then we just need to print the variable. Look at the full code for a clear idea:
int letmego; void setup() { Serial.begin(9600); while (Serial.available() == 0); letmego = Serial.parseInt(); Serial.print("Your input is: "); Serial.println(letmego); } void loop() { }
The output of the preceding code will be similar to the following screenshot, where I input on serial monitor 255:

If you want to take the input of a double number, all you need to change is the Serial.parseInt()
function, to Serial.parseFloat()
. Look at the following code, with output, where the input was 123.57:
float letmego; void setup() { Serial.begin(9600); while (Serial.available() == 0); letmego = Serial.parseFloat(); Serial.print("Your input is: "); Serial.println(letmego); } void loop() { }

If you want to read a character using the serial monitor, you need change the Serial.parseFloat()
function into Serial.read()
and set our variable as a character. Look at the following program, with output, where the input from the serial monitor was A:
char letmego; //data type changed void setup() { Serial.begin(9600); while (Serial.available() == 0); letmego = Serial.read(); //function changed Serial.print("Your input is: "); Serial.println(letmego); } void loop() { }

If you want to take an input of full string, then all you need to do is change the data type into String and change the Serial.read()
function to Serial.readString()
. Look at the following example, with output, where the input was To be or not to be, that is the question:
String letmego; void setup() { Serial.begin(9600); while (Serial.available() == 0); letmego = Serial.readString(); Serial.print("Your input is: "); Serial.println(letmego); } void loop() { }

Mathematical operations
Let x = 89, y = 98 and z = 45. What is the sum of three variables? You can sum up the values of x, y, and z and tell me the number. But since we have been learning C for Arduino, let's go a little further. We can do these kinds of mathematical operations using Arduino IDE - not just the easy mathematical operations, but far more complex mathematics can be solved using C for Arduino IDE.
Before going any further, let's learn about some arithmetic symbols used in Arduino programming. They are also called arithmetic operators:
Addition
By definition, addition sums two or more numbers. In C for Arduino, addition will do the same. This operation will add two or more numbers and store them in a variable. Let's look at an example.
We started the Mathematical operations section with a question to sum up three variables (x = 89, y = 98, and z = 45). Let's find the sum using Arduino programming.
We have three numbers. All numbers here are integers. We can assign them to be integer variables as follows:
int x = 89; int y = 98; int z = 45;
Now store them in another integer variable, say, the variable is sum:
int sum;
Store the sum of the variables (x, y, and z) into the variable sum, as follows:
sum = x+ y+ z;
Note
The addition/subtraction/pision/multiplication will be integers or double, if the operands are integers or double, respectively. Say, if the operands are integers, the output after the mathematical operation will also be integers. If they were double, the output will also be double.
Now print the variable sum on the serial monitor:
Serial.print(sum);
The full code and output will be as follows:
int x = 89; int y = 98; int z = 45; int sum; void setup() { Serial.begin(9600); sum = x=y+z; Serial.print("The sum of x, y and z is: "); // This line was written without any purpose. Just to fancy the output. Serial.print(sum); } void loop() { }

Now if you had 10 variables to sum up, I'm sure you could find the sum of them. Consider a problem: You have 23 pens, 25 pencils, 56 books, 32 apples, 89 oranges, 45 erasers, 87 bananas, and 98 chocolates. Make a list of two categories - food and non-food - and print the total number of food and non-food items using arithmetic operations.
The preceding problem has four foods (apples, oranges, bananas, and chocolates). So, we will sum them up and store them in a variable called food
. For non-foods, we have pens, pencils, books, and erasers. We will store the sum inside another variable, called non_food
.
So, our variables should be declared as follows:
int pens = 23; int pencils = 25; int books = 56; int apples =32; int oranges = 89; int erasers = 45; int bananas = 87; int chocolates = 98; int food; int non_food;
Now, let's assign the food
and non_food
variables with proper item values:
food = apples + oranges + bananas + chocolates; non_food = pens + pencils + books + erasers;
Finally, we will print both the variables. The full code of the program and output is as follows:
int pens = 23; int pencils = 25; int books = 56; int apples = 32; int oranges = 89; int erasers = 45; int bananas = 87; int chocolates = 98; int food; int non_food; void setup() { Serial.begin(9600); food = apples + oranges + bananas + chocolates; non_food = pens + pencils + books + erasers; Serial.print("You have "); Serial.print(food); Serial.println(" food items"); Serial.print("You have "); Serial.print(non_food); Serial.print(" non food items"); } void loop() { }

You can declare a variable in one, also; say you need to declare three double variables (num1
, num2
, and num3
). You can declare these as follows:
double num1, num2, num3;
This is similar to the following declaration:
double num1; double num2; double num3;
You can also declare any type of data type on a single line. If your variables have something assigned to them, you can write them as follows (where all are float and have the values num1 = 3.7, num2 = 87.9, and num3 = 8.9): float num1 = 3.7, num2 = 87.9, num3 = 8.9;
Subtraction
We can subtract a number from another number. By subtraction, we mean the difference between two numbers. From your math class, you might remember that we subtract the smaller number from the large number. Let's look at an example.
Say you have 649 red LEDs (Light Emitting Diodes) and 756 blue LEDs. You have used 123 red LEDs and 87 blue LEDs on a project. How many LEDs do you have now?
Let's declare our variables first:
int initialRedLED = 649, initialBlueLED = 756, spentRedLED = 123, spentBlueLED = 87, finalTotalLEDs, finalRedLED, finalBlueLED; // We have declared all our variables in one line.
We now find the difference between initial and spent LEDs, and store them in another two variables (finalRedLED
and finalBlueLED
) as follows:
finalRedLED = initialRedLED - spentRedLED; //to find the difference finalBlueLED = initialBlueLED - spentBlueLED; //to find the difference
Now print our variables finalRedLED
and finalBlueLED
.
To get the total LEDs left, we need to add the finalRedLED
and finalBlueLED
, and store them on another variable, finalTotalLEDs
, and print finalTotalLEDs
. So, our final code and output will be as follows:
int initialRedLED = 649, initialBlueLED = 756, spentRedLED = 123, spentBlueLED = 87, finalTotalLEDs, finalRedLED, finalBlueLED; void setup() { Serial.begin(9600); finalRedLED = initialRedLED - spentRedLED; finalBlueLED = initialBlueLED - spentBlueLED; finalTotalLEDs = finalRedLED + finalBlueLED; Serial.print("Initial Blue LEDs = "); Serial.println(initialBlueLED); Serial.print("Initial Red LEDs = "); Serial.println(initialRedLED); Serial.print("Final Red LEDs = "); Serial.println(finalRedLED); Serial.print("Finalt Blue LEDS = "); Serial.println(finalBlueLED); Serial.print("Total LEDs finally = "); Serial.print(finalTotalLEDs); } void loop() { }

Exercise
- Find the difference between 3.0×108 and 2.9×108 (hint: double x = 3.0E8 and double y = 2.9E8).
- Find the difference between 23 January 1960 and 4 July 2020 (hint: take three variables for day, month, and year for the first date, and for the second date, do the same. Use a numerical format for the month, that is, January is 1, February is 2, March is 3, and so on).
- Can you write the output of the following code?
void setup() { Serial.begin(9600); Serial.write(65); Serial.write(114); Serial.write(100); Serial.write(117); Serial.write(105); Serial.write(110); Serial.write(111); Serial.write(33); } void loop() { }
Multiplication
If you want to multiply a number with another number, you can do it using C for Arduino.
Let's consider another scenario. Suppose you have four books. Each book has 850 pages on average. You want to find the total number of pages of the book. You can simply add 850 four times, or you can multiply 850 by 4. That will be the number of pages. In C for Arduino, we will first declare a few variables, as follows:
int numberOfBooks = 4, numberOfPages = 850, totalPageNumbers;
Inside out setup function, we will do the calculations as follows:
totalPageNumbers = numberOfBooks * numberOfPages;
Then we will print the variable, totalPagenumbers
. The full code, with output, will be as follows:
int numberOfBooks = 4, numberOfPages = 850, totalPageNumbers; void setup() { Serial.begin(9600); totalPageNumbers = numberOfBooks * numberOfPages; Serial.print("Total books numbers "); Serial.println(numberOfBooks); Serial.print("Page numbers per book "); Serial.println(numberOfPages); Serial.print("Total numbers of pages "); Serial.println(totalPageNumbers); } void loop() { }

Let's write a program that can find the total number of pages of a number of books, input by the user.
Say we will input the number of books, and pages of each book. Our program will print the total number of pages.
From the input section of this chapter, you already know how you can take input of an integer. Now we will do another thing, to take integers, store inside a variable, and use them for calculations.
Declare three variables as follows:
int numberOfBooks, numberOfPages, totalPageNumber;
Take input of the number of book by the following line:
numberOfBooks = Serial.parseInt();
Before that, use a delay()
function with a time parameter. The Arduino board will wait that much time for the input on the serial monitor. Then take another variable input the same as the previous one. The code will be as follows:
int numberOfBooks, numberOfPages, totalPageNumber; void setup() { Serial.begin(9600); Serial.print("Input Number of books\n"); delay(1000); // Arduino will wait 1 sec for the input numberOfBooks = Serial.parseInt(); Serial.print("Input Number of pages\n"); delay(1000); // Arduino will wait 1 sec for the input numberOfPages = Serial.parseInt(); totalPageNumber = numberOfBooks * numberOfPages; Serial.print("Total Number of pages\n"); Serial.print(totalPageNumber); } void loop() { }
Let's look at the output with an input of 30 (number of books) and 560 (number of pages per book) The output will look as follows:

You can practice the following exercise at home:
- Take input of three float variables (u, t, and a) and find the distance (s) from the following equation:
S = ut+
at2
(Hints: t2 is equivalent to t*t in C. The whole equation will be s = u*t+0.5*a*t*t. To take input of a float, use
Serial.parseFloat()
) - Take inputs of three numbers and one character, and print them onto one line.
- Take input of two double numbers and print them onto two lines.
- Take input of an asterisk (*) and print as follows:
a. * ** *** b. *** ** * c. * ** ***
- Take input of two characters (V and A) and two integers (0 and 1), and print them as follows:
a. VA01 b. 01AV c. 0AV1 d. 000V e. VVVV f. VAVAVA
Division
We can pide a number with another, but we need to know the basic rules of pision in C.
Say we will pide 30 with 5. Since both are integers and 30 is totally pisible by 5, there will be no problem with the output. But if we wanted to pide 30 with 7, we will get 4.28 if we declare all the variables as float. If we declare our variables as integers, then our result will be 4. Look at the examples in the following screenshots.
If we declare our variables as integers and the first number is totally pisible by the second number, the output will be as follows:

If we declare our variables as integers and the first number is not totally pisible by the second number, the output will be as follows:

If we declare our variables as floats and the first number is totally pisible by the second number, the output will be as follows:

If we declare our variables as floats and the first number is not totally pisible by the second number, the output will be as follows:

So, we will have to be careful in finding the result of a pision. We will follow the last method, where we used all the variables to be floats to avoid any wrong answers.
Let's look at an example.
Suppose your electronics shop has 100 Arduinos that cost a total of $500 and you sold 20 Arduinos ($6.75 per Arduino). Find out the profit.
Now, define our variables:
float initialArduinos = 100.0, initialTotalPrice = 500.0, numberOfSell = 20.0, sellingPrice = 6.75, costPrice, totalSellingPrice, totalCostPrice, totalProfit;
Now it's time for some simple mathematics:
costPrice = initialTotalPrice / initialArduinos; // found per Arduino price Serial.print("Each arduino cost $"); Serial.println(costPrice); totalCostPrice = costPrice * numberOfSell; // found total cost price Serial.print("Total cost price for 20 Arduinos $"); Serial.println(totalCostPrice); totalSellingPrice = numberOfSell * sellingPrice ; // found total selling price Serial.print("Total selling price for 20 Arduinos $"); Serial.println(totalSellingPrice); totalProfit = totalSellingPrice - totalCostPrice; // found the profit for 20 arduinos. Serial.print("The total profil is $"); Serial.println(totalProfit);
The output of the code will be as follows:

Modulus
The modulus operator finds out the remainder. Say you want to pide 25 by 4. What will your remainder be? 4×6 =24. So, if we pide 25 by 4, we will get the result 4, and the remainder will be 1.
In C for Arduino, a modulus operator is the % symbol. The representation of 25 modulus 4 is 25%4, which is equal to 1. So, we can write 25%4 = 1.
Let's look at an example:
Find out the remainders if 623 is pided by 4, 5, 6, 7, 8, and 9.
Let's declare our variables.
int myNumber = 623, remainder;
Now find the remainder:
Remainder = myNumber % 4; // or 5/6/7/8/9
Now print the remainder. So, the total code, with output, will look as follows:
int myNumber = 623, reminder; void setup() { Serial.begin(9600); reminder = myNumber % 4; Serial.print("reminder by 4 is "); Serial.println(reminder); reminder = myNumber % 5; Serial.print("reminder by 5 is "); Serial.println(reminder); reminder = myNumber % 6; Serial.print("reminder by 6 is "); Serial.println(reminder); reminder = myNumber % 7; Serial.print("reminder by 7 is "); Serial.println(reminder); reminder = myNumber % 8; Serial.print("reminder by 8 is "); Serial.println(reminder); reminder = myNumber % 9; Serial.print("reminder by 9 is "); Serial.println(reminder); } void loop() { }

There are more mathematical operations. We will learn about them as needed, in the following chapters.
Arrays
C for Arduino provides users the capability to design a set of the same data type. This functionality is called an array. An array is a collection of the same data type into one variable.
Let's consider the following code:
int x; void setup() { x = 23; x = 78; Serial.begin(9600); Serial.print(x); } void loop() { }
Can you tell me the output of this code?
x was defined as an integer as a global variable. Later, inside the setup()
function, it was first assigned to number 23, and second to 78. No doubt the output will be 78, because it was defined later and C reads the program line by line, or from top to bottom. To avoid the complexity, we use arrays.
Say we need to store 100 same-type variables and use them later. We can do this in two ways:
- Take 100 variables and assign values to them
- Take a single variable and store all values to it
The second option is easy and less time consuming. Now, the burning question is, how can we declare an array? Well, it's simple. Let's look at an example first:
int myArray[5] = {3,5,7,8,3};
Look at the following screenshot:

myArray is the name of the variable, and inside the brackets, there is 5, which is the limit of the array. This means how many variables the array can contain. This number fits your need; you can declare any number you want. On the right-hand side, there are five numbers (as our array can contain five variables) inside curly brackets, separated by commas. Each number has an index number by which we will call our variables. The index numbers start from 0. So, our first number (which is 3) can be called by myArray[0]
. If we print myArray[0]
, we will get 3
. Similarly, if we call myArray[1]
, we will get 5
, and so on. Let's check it with the following code:
int myArray[5] = {3, 5, 7, 8, 3}; void setup() { Serial.begin(9600); Serial.print("Index number is 0 and the value is "); Serial.println(myArray[0]); Serial.print("Index number is 1 and the value is "); Serial.println(myArray[1]); Serial.print("Index number is 2 and the value is "); Serial.println(myArray[2]); Serial.print("Index number is 3 and the value is "); Serial.println(myArray[3]); Serial.print("Index number is 4 and the value is "); Serial.println(myArray[4]); } void loop() { }

The final index number is N-1, where n is the number inside brackets []. So, we cannot call the index number N or greater than N. We cannot even call a negative index number . What would happen if we called them? Let's look at the following code and output:
int myArray[5] = {3, 5, 7, 8, 3}; void setup() { Serial.begin(9600); Serial.print("Index number is -1 and the value is "); Serial.println(myArray[-1]); //negative index number Serial.print("Index number is 5 and the value is "); Serial.println(myArray[5]); // N index number Serial.print("Index number is 6 and the value is "); Serial.println(myArray[6]); // Greater than N index number. } void loop() { }

From the output, you can see that, when we called an index which is less than N, we get a number. For now, we call it a garbage number. It is not in our array. This is because the microcontroller reserves its memory for the data types. If we call the memory location, we get something stored in the location. If nothing is there, we get zero. That is why, when we called the index equal to N, we got 0. There is nothing in the memory. The same thing happened when we called the index greater than N.
The data type of an array can be anything (integers, double, float, character, bytes, Boolean, and so on). Let's look at another example.
Suppose a class has 20 students. You took an exam worth 50 marks and you need to store all the marks in your program according to roll numbers. We know that it is not wise to make 20 variables and call them one by one when necessary, as long as we can use an array. So, let's use an array and find the numbers of roll number 3, 7, and 16. First, declare our variable. The marks cannot be integers, so we take our variable as float:
float marks[20] = {45.5, 23.2, 0, 34, 45.9, 50, 12, 33, 34.5,0, 23.9, 15, 18, 17.4, 18.3, 10, 4, 9, 34.8, 4.5};
Now, we print the array according to our index number.
- For the roll 3, the index number is 3-1 = 2.
- For the roll 7, the index number is 7-1 = 6.
- For the roll 16, the index number is 16-1 = 15.
The full code will be as follows:
float marks[20] = {45.5, 23.2, 0, 34, 45.9, 50, 12, 33, 34.5, 0, 23.9, 15, 18, 17.4, 18.3, 10, 4, 9, 34.8, 4.5};
void setup() { Serial.begin(9600); Serial.print("Mark obtained by roll 3 is "); Serial.println(marks[2]); //roll number 3's marks Serial.print("Mark obtained by roll 7 is "); Serial.println(marks[6]); //roll number 7's marks Serial.print("Mark obtained by roll 16 is "); Serial.println(marks[15]); //roll number 16's marks } void loop() { }
The output of the code will be as follows:

Let's do the same thing with two arrays containing the roll numbers of the students. This time, we will print the marks obtained by the students and their roll numbers for roll 1, 4, 8, 7, and 9.
Our second array will be defined as follows:
int roll [20] = {1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20};
Now we will print the roll numbers and marks according to the following code:
float marks[20] = {45.5, 23.2, 0, 34, 45.9, 50, 12, 33, 34.5, 0, 23.9, 15, 18, 17.4, 18.3, 10, 4, 9, 34.8, 4.5}; int roll [20] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20}; void setup() { Serial.begin(9600); Serial.print("Mark obtained by roll "); Serial.print(roll[0]); //this is roll number 1, since the first element of the array. Serial.print(": "); Serial.println(marks[0]); //roll number 1's marks Serial.print("Mark obtained by roll "); Serial.print(roll[3]); //this is roll number 4, since the first element of the array. Serial.print(": "); Serial.println(marks[3]); //roll number 4's marks Serial.print("Mark obtained by roll "); Serial.print(roll[7]); //this is roll number 8, since the first element of the array. Serial.print(": "); Serial.println(marks[7]); //roll number 1's marks Serial.print("Mark obtained by roll "); Serial.print(roll[6]); //this is roll number 7, since the first element of the array. Serial.print(": "); Serial.println(marks[6]); //roll number 1's marks Serial.print("Mark obtained by roll "); Serial.print(roll[8]); //this is roll number 9, since the first element of the array. Serial.print(": "); Serial.println(marks[8]); //roll number 9's marks } void loop() { }
Let's check the output:

Look at the index numbers we used. I hope you understand the meaning of index number now.
If you want to make an array of characters (a, e, i, o, and u), your array will look as follows:
char vowel[5] = {'a', 'e', 'i', 'o', 'u'};
If you call by the third index, you will get o
, since by the third index, we mean the fourth position of the array list. Let's look at the output:

Each character should have single quotes, as we declared before. We will discuss how you can declare String
arrays later.
Finally, we can say that an array is a collection of similar elements. The similar elements can be all integers
, floats
, doubles
, characters
, bytes
, and so on.
We have learned about single-dimensional arrays. There are also multi-dimensional arrays. Let's look at an example.
You may have heard of a matrix in your math class. In the following code, A, B, and C are three matrices:

- The number of rows and columns of matrix A is 3×3 (read 3 by 3)
- The number of rows and columns of matrix B is 2×2 (read 2 by 2)
- The number of rows and columns of matrix C is 2×3 (read 2 by 3)
So, the total elements can be found by multiplying the rows and columns. The convention is writing the numbers of the rows first, followed by the numbers of the columns, when we write the dimensions of a matrix (that is, rows × columns) The B matrix in C for Arduino can be written as follows:
int B[2][2] ={{2,5},{6,7}};
If we want to call the first element of the first row, we can call it by printing the following array with 0
and 0
indexes:
Serial.print(B[0][0]);
The second element of the first row is as follows:
Serial.print(B[0][1]);
The first element of the second array is as follows:
Serial.print(B[1][0]);
The second element of the second row is as follows:
Serial.print(B[1][1]);
Let's call the whole matrix in a program; the code will be as follows:
int B[2][2] = {{2, 5}, {5, 7}}; void setup() { Serial.begin(9600); Serial.print(B[0][0]); Serial.print(" "); Serial.println(B[0][1]); Serial.print(B[1][0]); Serial.print(" "); Serial.print(B[1][1]); } void loop() { }
The output of the code will be as follows:

Exercise
- Make a list of the following food items, with the prices next to them. If a customer buys five pieces of food item c. on the list and five pieces of food item e. on the list, calculate the total money he would need to buy the food items:
- Sandwiches ($2.90)
- Burgers ($4.90)
- Pizzas ($9.99)
- Soft Drinks ($1.50)
- Beer ($4.99)
Your output should look as follows:
****The customer receipt**** Food Name Quantity Price Total Sandwiches 0 2.90 0 Burgers 0 4.90 0 Pizzas 5 9.99 49.95 Soft Drinks 0 1.50 0 Beer 5 4.99 24.95 Total: $ 74.90
(Hints: Make two array lists for the quantity and price of each items. Then multiply the array with the correct index number. You will find the total prices. Then, sum up all the prices.)
- Assume you have the following array:
char symbol [10] = {'!', '@', '#', '$', '%', '&', '*', '+', '=', '_'};
Now write the following programs for the following outputs:@
@ #@# $@#@$@#@ &$@#@$@#@ !!!!!!!!!!*!!!!!!!!! %%%%****%%%% *^E^&!$#@(
- Write a program for the following output using modulus, arrays, and other mathematical operators:
(Hints: Take two arrays. One is five characters and another is five integers. Then find the modulus of the number arrays by 4. Finally, print them.)
Print the sum of the following matrices:

If we used loops for our program, the program would have been much easier. We will learn about loops in the next chapter (Blinking with Operations and Loops).