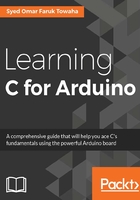
Variables
Variables are used to store data in programming languages. You might remember from your algebra class that, The number of chocolates is x, where we didn't know the value of x, but after the math was done, we found the value of x. Let's make it clear by giving an example. Consider a scenario: A boy has 23 pencils; he buys 13 pencils. How many pencils does he have now?
It's simple. Just sum up all the numbers. But let's try to do it using a variable. We can solve it as follows:
The number of pencils the boy has now is x:
So, x = 23+13 Or, x = 46
We have found the value of x, which is the total number of pencils the boy has now.
Have you noticed we stored the number of pencils on the variable x? Yes, this is what we do with variables.
In C programming, we often need to declare variables inside our code. During the C program execution, an entity that may vary is called a variable. There are a few rules for declaring a variable name. We cannot name our variables however we want, but aside from the following rules, we can use any variable name we want. The rules are:
You cannot declare a variable that starts with a number. For example, 1stNumber, 007User, 2ndName, and so on cannot be declared as variables in C.
Your variable's name should not contain any symbols. This means that &name, %testVar, #time, and so on cannot be declared and used in C programming as variable names. That said, the dollar sign ($) and underscore (_) are fine to be used in a variable name. However, in Arduino IDE, we cannot use a dollar sign ($) in the variable name. This will show error.
Your variables must not have blank spaces, which means, my test, his name, the cost , and so on are not valid variables in C.
The variables cannot contain commas or periods. My.variable, my, age , and so on are not valid.
Other than the preceding rules, you should also remember that your variable name can have up to 247 characters. On some compilers, it is limited to 31 characters. Since C programming is case sensitive, myname and MYNAME are different variables.
Exercise
Can you tell me which of the following variable names is valid and why?
_number, $name, varItems, 0isScore, -number, look@me, ~var, TimeLimit, my1stclass, weeknumber1, december23, 23october, like+ride.
Note
Aside from the rules and length limit of a variable name, there is one thing we should remember. We cannot use reserved keywords as our variable names. There are a few reserved keywords in C that we cannot use for declaring variables. The reserved keywords are: auto, break, case, char, const, continue, default, do, double, else, enum, extern, float, for, goto, if, int, long, register, return, short, signed, sizeof, static, struct, switch, typeof, union, unsigned, void, volatile, while.